Java Exercises: Accepts a string from the user and check whether the string is correct or not
Java Basic: Exercise-244 with Solution
Write a Java program which accepts a string from the user and check whether the string is correct or not.
The conditions for getting the "correct answer" are:
a) There must be only three characters X, Y, and Z in the string, and no other characters.
b) Any string of any form such as aXYZa can get the "correct answer", where a is either an empty string or a string consisting only of the letter X;
c) If aXbZc is correct, aXbYZca is also correct, where a, b, c are either empty strings or a string consisting only of the letter X.
Sample Solution:
Java Code:
import java.util.PriorityQueue;
import java.util.Scanner;
public class Main {
static class Dic implements Comparable<Dic>{
String moji;
int page;
Dic(String moji, int page){
this.moji=moji;
this.page=page;
}
public int compareTo(Dic d) {
if(this.moji.equals(d.moji)) {
return this.page-d.page;
}
else {
return this.moji.compareTo(d.moji);
}
}
}
public static void main(String[] args) {
try(Scanner sc = new Scanner(System.in)){
PriorityQueue<Dic> pq=new PriorityQueue<>();
System.out.println("Input pairs of a word and a page number:");
while(sc.hasNextLine()) {
String str=sc.nextLine();
String[] token=str.split(" ");
String s=token[0];
int n=Integer.parseInt(token[1]);
pq.add(new Dic(s, n));
}
String pre="";
System.out.println("\nWord and page number in alphabetical order:");
while(!pq.isEmpty()) {
Dic dic=pq.poll();
if(dic.moji.equals(pre)) {
System.out.print(" "+dic.page);
}
else if(pre.equals("")) {
System.out.println(dic.moji);
System.out.print(dic.page);
}
else {
System.out.println();
System.out.println(dic.moji);
System.out.print(dic.page);
}
pre=dic.moji;
}
System.out.println();
}
}
}
Sample Output:
Input a string: XYZ Correct format..
Pictorial Presentation:
Flowchart:
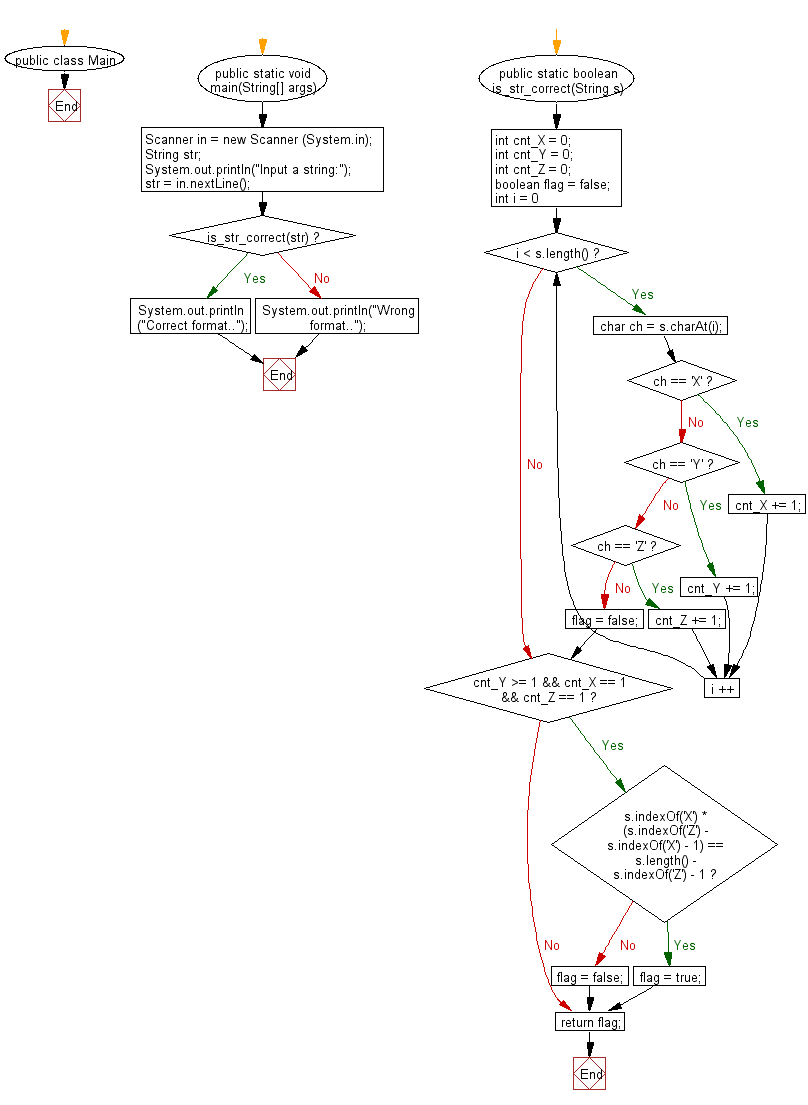
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program which reads a list of pairs of a word and a page number, and prints the word and a list of the corresponding page numbers.
Next: Write a Java program which accepts students name, id, and marks and display the highest score and the lowest score.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework