Java Exercises: Reads a date and prints the day of the date
Java Basic: Exercise-225 with Solution
Write a Java program to that reads a date (from 2004/1/1 to 2004/12/31) and prints the day of the date. Jan. 1, 2004, is Thursday. Note that 2004 is a leap year.
Pictorial Presentation:
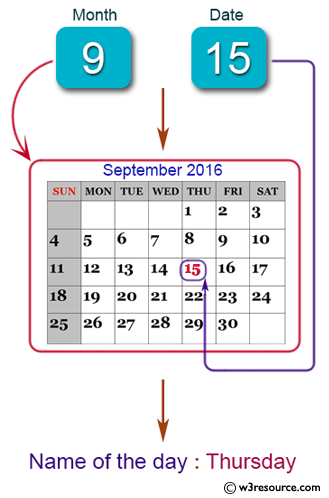
Sample Solution:
Java Code:
import java.util.*;
class Main {
static int days[] = {31, 29, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
static String name[] = {"Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday"};
public static void main(String args[]){
Scanner in = new Scanner(System.in);
System.out.println("Input month and date(o o to exit)");
for(;;){
int m=in.nextInt(), d=in.nextInt();
if(m==0&&d==0) break;
System.out.println(solve(m,d));
}
}
static String solve(int month, int date){
int cur = 3;
System.out.println("Name of the day:");
for(int i=0; i<month-1; i++) cur += days[i];
cur += date-1;
return name[cur%7];
}
}
Sample Output:
Input month and date(o o to exit) 9 15 Name of the day: Wednesday
Flowchart:
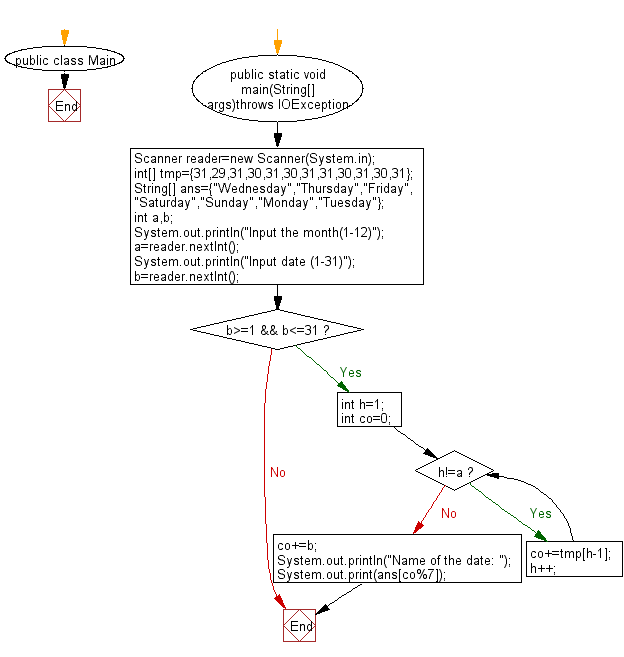
Java Code Editor:
Contribute your code and comments through Disqus.
Previous:Write a Java program to test the followings.
Next: Write a Java program to print mode values from a given a sequence of integers. The mode value is the element which occurs most frequently. If there are several mode values, print them in ascending order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework