Java Exercises: Compute the amount of the debt in n months
Java Basic: Exercise-215 with Solution
Write a Java program to compute the amount of the debt in n months. The borrowing amount is $100,000 and the loan adds 4% interest of the debt and rounds it to the nearest 1,000 above month by month.
Input:
An integer n (0 ≤ n ≤ 100).
Sample Solution:
Java Code:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
System.out.println("Input number of months:");
int n = s.nextInt();
double c = 100000;
for(int i = 0; i < n; i++) {
c += c*0.04;
if(c % 1000 != 0) {
c -= c % 1000;
c += 1000;
}
}
System.out.println("\nAmount of debt: ");
System.out.printf("%.0f\n",c);
}
}
Sample Output:
Input number of months: 6 Amount of debt: 129000
Flowchart:
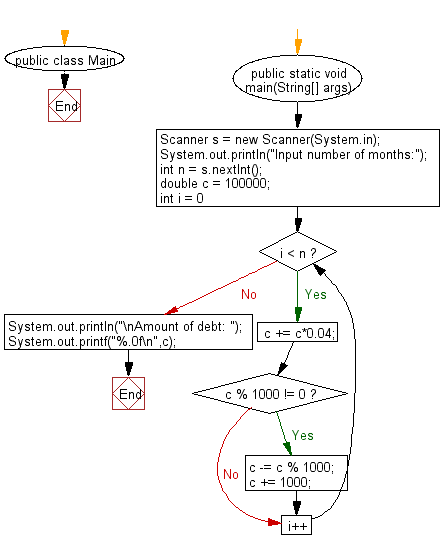
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program which solve the equation. Print the values of x, y where a, b, c, d, e and f are specified.
Next: Write a Java program which reads an integer n and find the number of combinations of a,b,c and d will be equal to n.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework