Java Exercises: Compute the digit number of sum of two given integers
Java Basic: Exercise-212 with Solution
Write a Java program to compute the digit number of sum of two given integers.
Input:
Each test case consists of two non-negative integers a and b which are separated by a space in a line. 0 ≤ a, b ≤ 1,000,000
Pictorial Presentation:
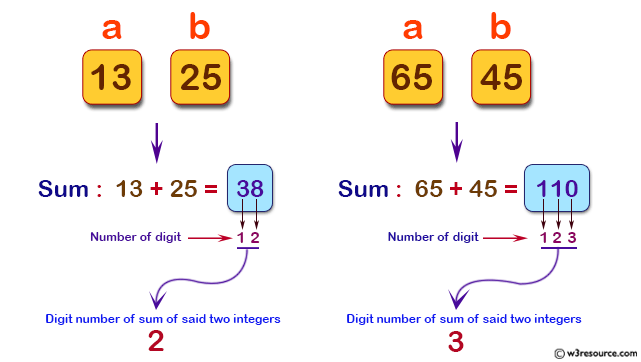
Sample Solution:
Java Code:
import java.util.*;
public class Main
{
public static void main(String[] args)
{
System.out.println("Input two integers(a b):");
Scanner stdIn = new Scanner(System.in);
int a = stdIn.nextInt();
int b = stdIn.nextInt();
int sum = a + b;
int count = 0;
while(sum != 0)
{
sum /= 10;
++count;
}
System.out.println("Digit number of sum of said two integers:");
System.out.println(count);
}
}
Sample Output:
Input two integers(a b): 13 25 Digit number of sum of said two integers: 2
Flowchart:
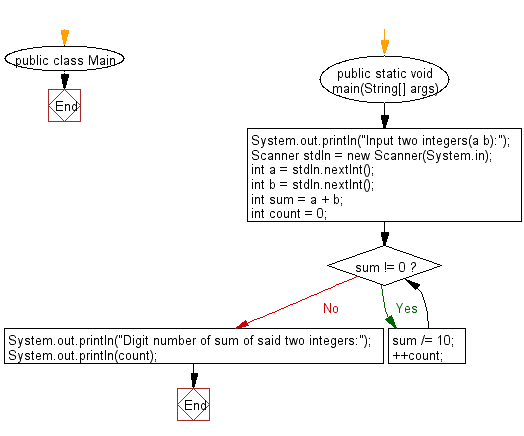
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to find heights of the top three building in descending order from eight given buildings.
Next: Write a Java program to check whether three given lengths (integers) of three sides form a right triangle. Print "Yes" if the given sides form a right triangle otherwise print "No".
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework