Java Exercises: Test whether there are two integers x and y
Java Basic: Exercise-191 with Solution
Write a Java program to test whether there are two integers x and y such that x^2 + y^2 is equal to a given positive number.
Pictorial Presentation:
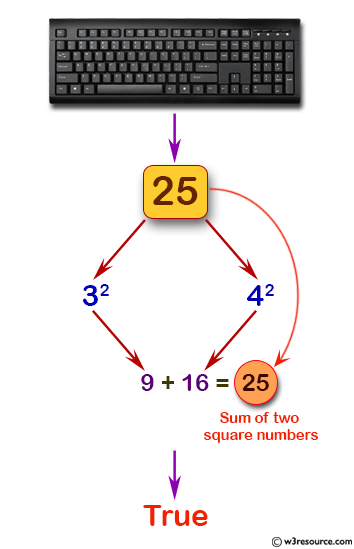
Sample Solution:
Java Code:
import java.util.*;
public class Solution {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
System.out.print("Input a positive integer: ");
int n = in.nextInt();
if (n>0)
{
System.out.print("Is "+n+" sum of two square numbers? "+sum_of_square_numbers(n));
}
}
public static boolean sum_of_square_numbers(int n) {
int left_num = 0, right_num = (int) Math.sqrt(n);
while (left_num <= right_num) {
if (left_num * left_num + right_num * right_num == n) {
return true;
} else if (left_num * left_num + right_num * right_num < n) {
left_num++;
} else {
right_num--;
}
}
return false;
}
}
Sample Output:
Input a positive integer: 25 Is 25 sum of two square numbers? true
Flowchart:
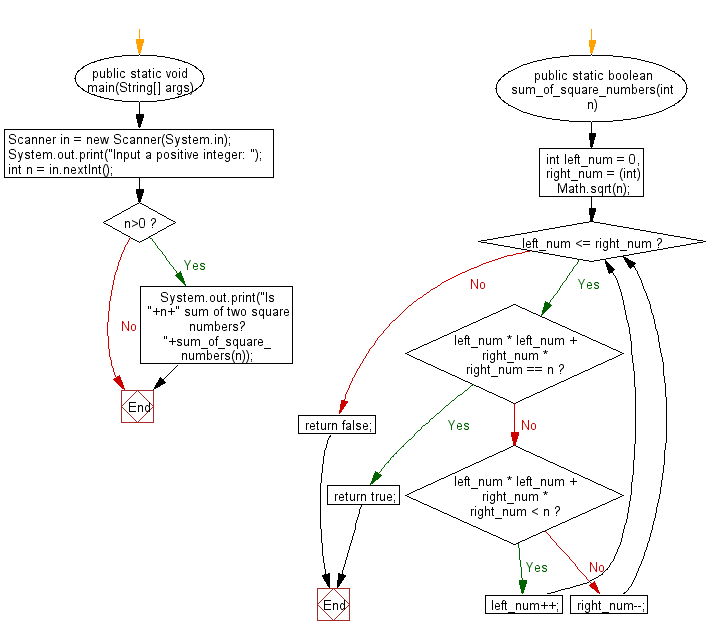
Java Code Editor:
Company: LinkedIn
Contribute your code and comments through Disqus.
Previous: Write a Java program to find the missing string from two given strings.
Next: Write a Java program to rearrange the alphabets in the order followed by the sum of digits in a given string containing uppercase alphabets and integer digits (from 0 to 9).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework