Java Exercises: Find the length of the longest consecutive sequence path of a given binary tree
Java Basic: Exercise-184 with Solution
Write a Java program to find the length of the longest consecutive sequence path of a given binary tree.
Note: The longest consecutive path need to be from parent to child.
Sample Binary tree:
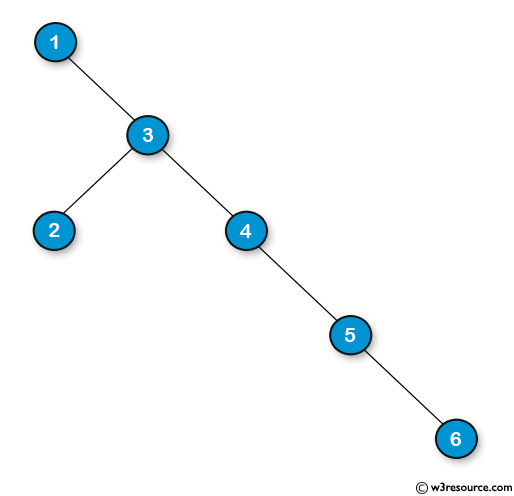
Result:

Sample Solution:
Java Code:
import java.util.*;
public class Solution {
public static void main(String[] args) {
TreeNode a = new TreeNode(1);
a.right = new TreeNode(3);
a.right.left = new TreeNode(2);
a.right.right = new TreeNode(4);
a.right.right.right = new TreeNode(5);
a.right.right.right.right = new TreeNode(6);
System.out.println("Length of the longest consecutive sequence path: "+longest_Consecutive(a));
}
public static int longest_Consecutive(TreeNode root) {
if (root == null) {
return 0;
}
int result = diffn(root, 1) + diffn(root, -1);
return Math.max(result, Math.max(longest_Consecutive(root.left), longest_Consecutive(root.right)));
}
private static int diffn(TreeNode tnode, int diff) {
if (tnode == null) {
return 0;
}
int left_depth = 0, right_depth = 0;
// check node
if (tnode.left != null && tnode.val - tnode.left.val == diff) {
left_depth = diffn(tnode.left, diff) + 1;
}
if (tnode.right != null && tnode.val - tnode.right.val == diff) {
right_depth = diffn(tnode.right, diff) + 1;
}
return Math.max(left_depth, right_depth);
}
}
Sample Output:
Length of the longest consecutive sequence path: 4
Flowchart:
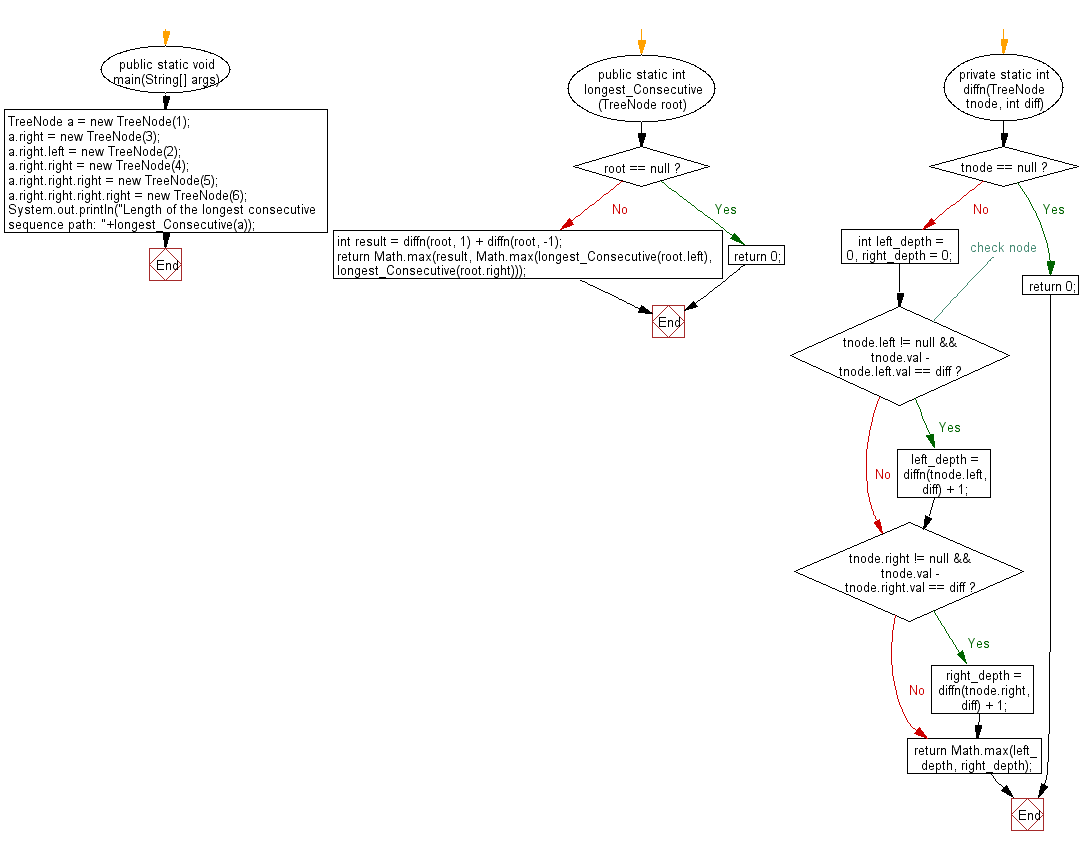
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to accept a positive number and repeatedly add all its digits until the result has only one digit.
Next: Write a Java program to check if two given strings are isomorphic or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework