Java Exercises: Find the length of the longest consecutive sequence of a given array of integers
Java Basic: Exercise-170 with Solution
Write a Java program to find the length of the longest consecutive sequence of a given array of integers.
Pictorial Presentation:
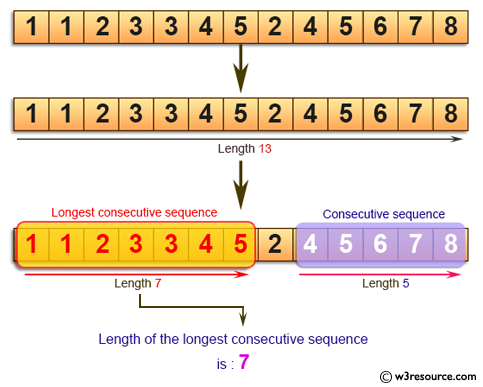
Sample Solution:
Java Code:
import java.util.*;
public class Solution {
public static int longest_sequence(int[] nums) {
if (nums == null) {
throw new IllegalArgumentException("Null array..!");
}
if (nums.length == 0) {
return 0;
}
boolean flag = false;
int result = 0;
int start = 0, end = 0;
for (int i = 1; i < nums.length; i++) {
if (nums[i - 1] < nums[i]) {
end = i;
} else {
start = i;
}
if (end - start > result) {
flag = true;
result = end - start;
}
}
if (flag)
{
return result + 1;
}
else
{
return result;
}
}
public static void main(String[] args) {
int[] nums = { 1, 1, 2, 3, 3, 4, 5, 2, 4, 5, 6, 7, 8, 9, 6, -1, -2 };
System.out.println("\nOriginal array: "+Arrays.toString(nums));
System.out.println(longest_sequence(nums));
}
}
Sample Output:
Original array: [1, 1, 2, 3, 3, 4, 5, 2, 4, 5, 6, 7, 8, 9, 6, -1, -2] 7
Flowchart:
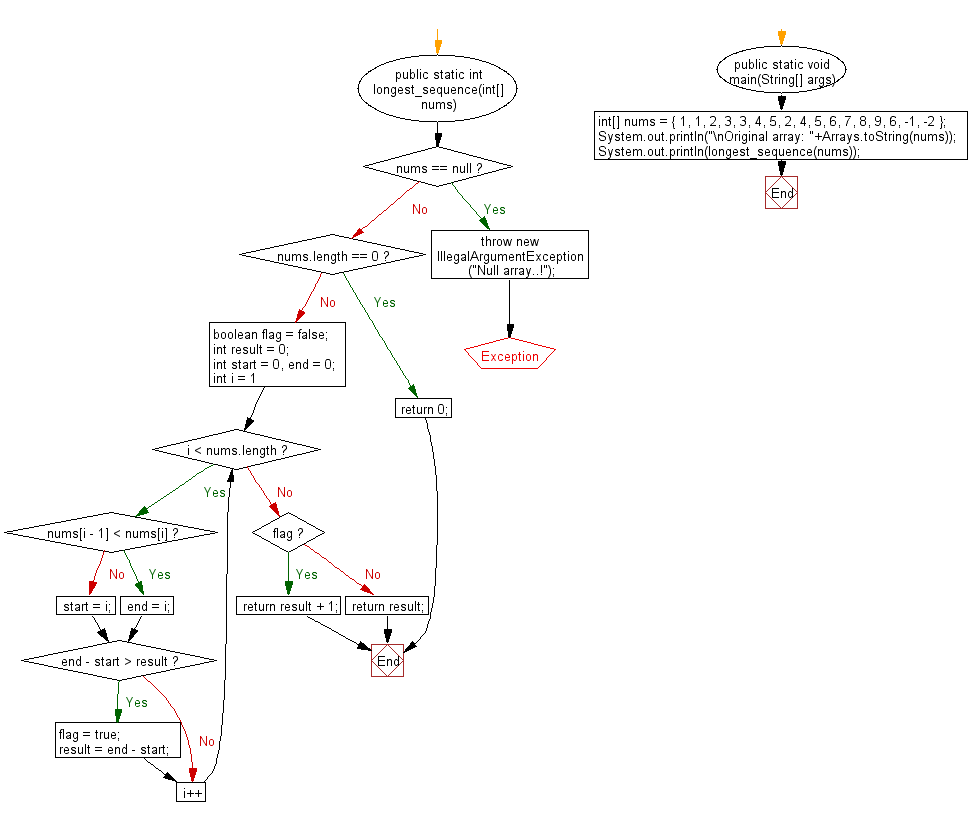
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to reverse the content of a sentence (assume a single space between two words) without reverse every word.
Next: Write a Java program to accept two string and test if the second string contains the first one.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework