Java Exercises: Compute the number of trailing zeros in a factorial
Java Basic: Exercise-112 with Solution
Write a Java program to compute the number of trailing zeros in a factorial.
Example
7! = 5040, therefore the output should be 1.
Pictorial Presentation:
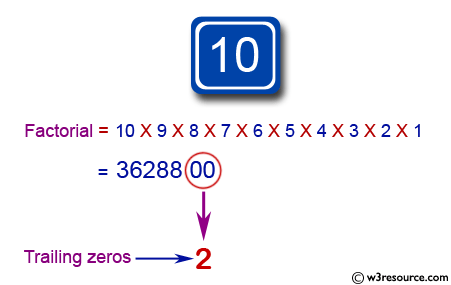
Sample Solution:
Java Code:
import java.util.Scanner;
public class Example112 {
public static void main(String[] arg)
{
Scanner in = new Scanner(System.in);
System.out.print("Input a number: ");
int n = in.nextInt();
int n1 = n;
long ctr = 0;
while (n != 0)
{
ctr += n / 5;
n /= 5;
}
System.out.printf("Number of trailing zeros of the factorial %d is %d ",n1,ctr);
System.out.printf("\n");
}
}
Sample Output:
Input a number : 5040 Number of trailing zeros of the factorial 5040 is 1258
Flowchart:
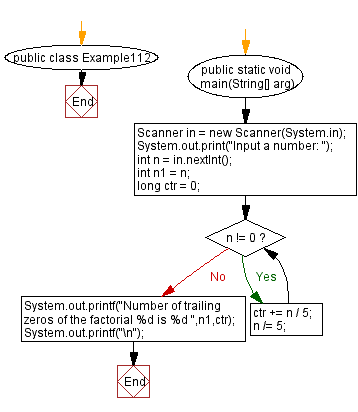
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to add two numbers without using any arithmetic operators.
Next: Write a Java program to merge two given sorted array of integers and create a new sorted array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework