Java Exercises: Check whether a given integer is a power of 4 or not
Java Basic: Exercise-110 with Solution
Write a Java program to check whether an given integer is a power of 4 or not.
Given num = 64, return true. Given num = 6, return false.
Pictorial Presentation:

Sample Solution:
Java Code:
import java.util.Scanner;
public class Example110 {
public static void main(String[] arg)
{
int test = 0;
Scanner in = new Scanner(System.in);
System.out.print("Input a positive integer: ");
int n = in.nextInt();
if (n < 1) {
System.out.print(Boolean.toString(false));
test = 1;
}
if ((n & (n - 1)) != 0) {
System.out.print(Boolean.toString(false));
test = 1;
}
if (test==0)
{
System.out.print(Boolean.toString((n & 0x55555555) != 0));
}
System.out.print("\n");
}
}
Sample Output:
Input a positive integer: 64 true
Flowchart:
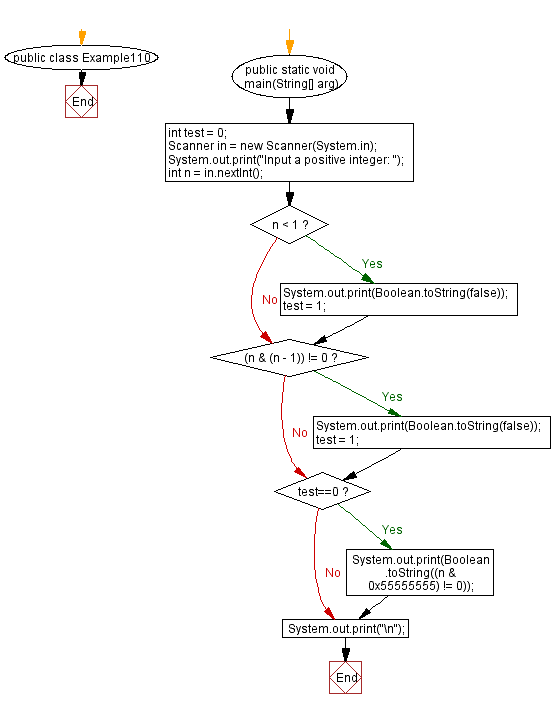
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to form a staircase shape of n coins where every k-th row must have exactly k coins.
Next: Write a Java program to add two numbers without using any arithmetic operators.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework