Java Array Exercises: Check if a sub-array is formed by consecutive integers from a given array of integers
Java Array: Exercise-57 with Solution
Write a Java program to check if a sub-array is formed by consecutive integers from a given array of integers.
Example:
Input :
nums = { 2, 5, 0, 2, 1, 4, 3, 6, 1, 0 }
Output:
The largest sub-array is [1, 7]
Elements of the sub-array: 5 0 2 1 4 3 6
Sample Solution:
Java Code:
import java.lang.Math;
import java.util.Arrays;
class solution
{
static boolean is_consecutive(int nums[], int i, int j, int min, int max)
{
if (max - min != j - i) {
return false;
}
boolean check[] = new boolean[j - i + 1];
for (int k = i; k <= j; k++)
{
if (check[nums[k] - min]) {
return false;
}
check[nums[k] - min] = true;
}
return true;
}
public static void find_Max_SubArray(int[] nums)
{
int len = 1;
int start = 0, end = 0;
for (int i = 0; i < nums.length - 1; i++)
{
int min_val = nums[i], max_val = nums[i];
for (int j = i + 1; j < nums.length; j++)
{
min_val = Math.min(min_val, nums[j]);
max_val = Math.max(max_val, nums[j]);
if (is_consecutive(nums, i, j, min_val, max_val))
{
if (len < max_val - min_val + 1)
{
len = max_val - min_val + 1;
start = i;
end = j;
}
}
}
}
System.out.println("The largest sub-array is [" + start + ", "
+ end + "]");
System.out.print("Elements of the sub-array: ");
for (int x = start; x <= end; x++)
{
System.out.print(nums[x]+" ");
}
}
public static void main (String[] args)
{
int[] nums = { 2, 5, 0, 2, 1, 4, 3, 6, 1, 0 };
System.out.println("Original array: "+Arrays.toString(nums));
find_Max_SubArray(nums);
}
}
Sample Output:
Original array: [2, 5, 0, 2, 1, 4, 3, 6, 1, 0] The largest sub-array is [1, 7] Elements of the sub-array: 5 0 2 1 4 3 6
Flowchart:
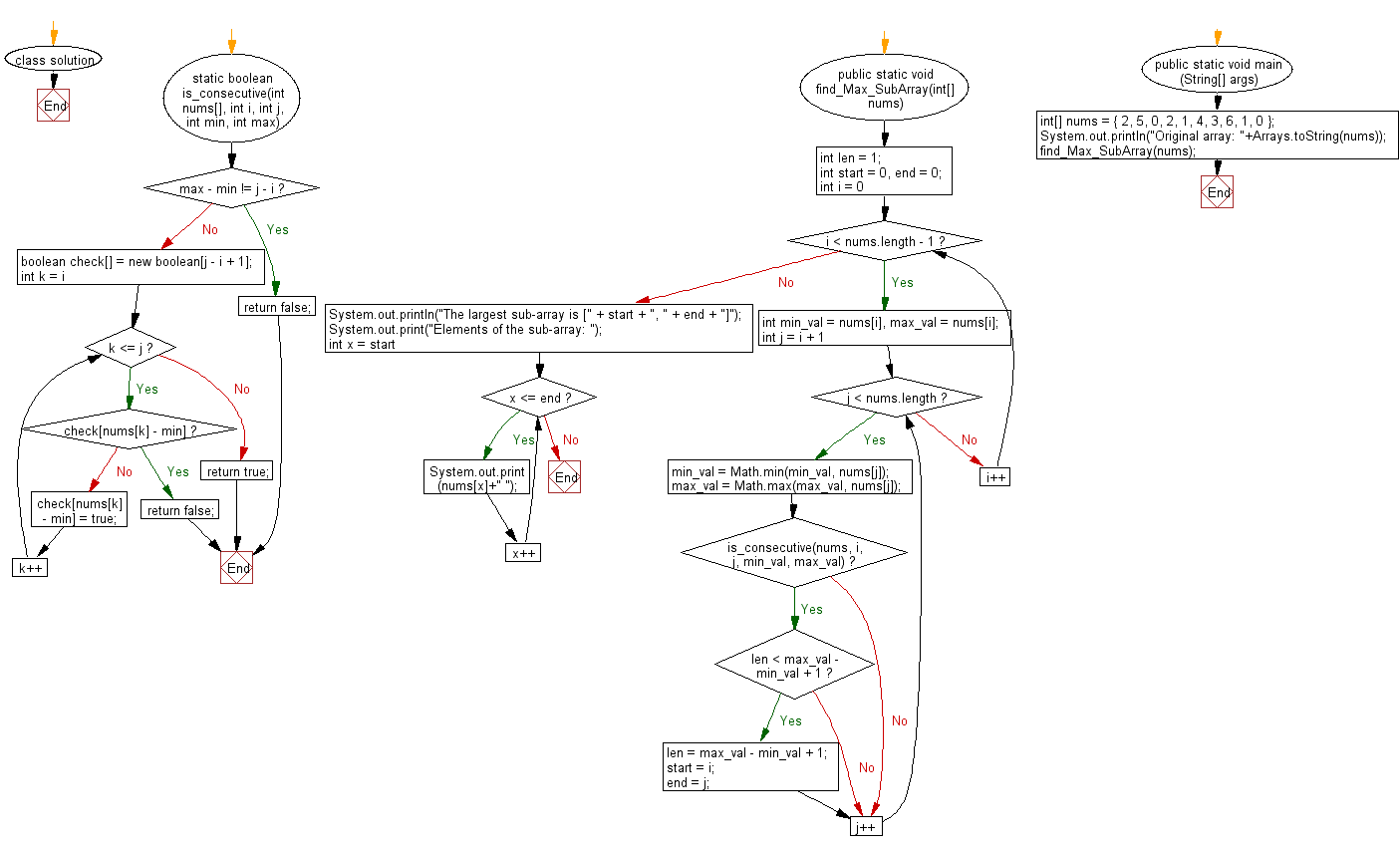
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to sort a given binary array in linear times.
Next: write a Java program to merge elements of A with B by maintaining the sorted order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework