Java Array Exercises: Check if a given array contains a subarray with 0 sum
Java Array: Exercise-54 with Solution
Write a Java program to check if a given array contains a subarray with 0 sum.
Example:
Input :
nums1= { 1, 2, -2, 3, 4, 5, 6 }
nums2 = { 1, 2, 3, 4, 5, 6 }
nums3 = { 1, 2, -3, 4, 5, 6 }
Output:
Does the said array contain a subarray with 0 sum: true
Does the said array contain a subarray with 0 sum: false
Does the said array contain a subarray with 0 sum: true
Sample Solution:
Java Code:
import java.util.Set;
import java.util.HashSet;
import java.util.Arrays;
class solution
{
public static Boolean find_subarray_sum_zero(int[] nums)
{
Set<Integer> set = new HashSet<>();
set.add(0);
int suba_sum = 0;
for (int i = 0; i < nums.length; i++)
{
suba_sum += nums[i];
if (set.contains(suba_sum)) {
return true;
}
set.add(suba_sum);
}
return false;
}
public static void main (String[] args)
{
int[] nums1= { 1, 2, -2, 3, 4, 5, 6 };
System.out.println("Original array: "+Arrays.toString(nums1));
System.out.println("Does the said array contain a subarray with 0 sum: "+find_subarray_sum_zero(nums1));
int[] nums2 = { 1, 2, 3, 4, 5, 6 };
System.out.println("\nOriginal array: "+Arrays.toString(nums2));
System.out.println("Does the said array contain a subarray with 0 sum: "+find_subarray_sum_zero(nums2));
int[] nums3 = { 1, 2, -3, 4, 5, 6 };
System.out.println("\nOriginal array: "+Arrays.toString(nums3));
System.out.println("Does the said array contain a subarray with 0 sum: "+find_subarray_sum_zero(nums3));
}
}
Sample Output:
Original array: [1, 2, -2, 3, 4, 5, 6] Does the said array contain a subarray with 0 sum: true Original array: [1, 2, 3, 4, 5, 6] Does the said array contain a subarray with 0 sum: false Original array: [1, 2, -3, 4, 5, 6] Does the said array contain a subarray with 0 sum: true
Flowchart:
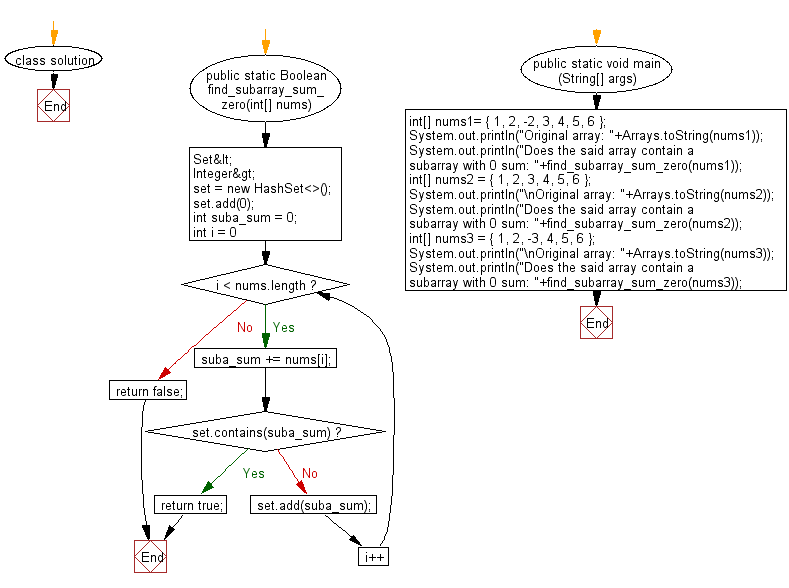
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to replace every element with the next greatest element (from right side) in a given array of integers.
Next: Write a Java program to print all sub-arrays with 0 sum present in a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework