Java Array Exercises: Test if an array contains a specific value
Java Array: Exercise-5 with Solution
Write a Java program to test if an array contains a specific value.
Pictorial Presentation:
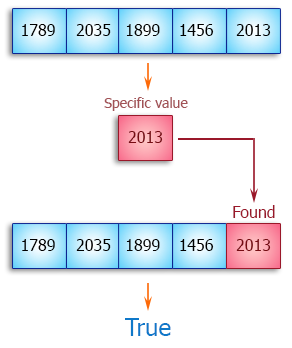
Sample Solution:
Java Code:
public class Exercise5 {
public static boolean contains(int[] arr, int item) {
for (int n : arr) {
if (item == n) {
return true;
}
}
return false;
}
public static void main(String[] args) {
int[] my_array1 = {
1789, 2035, 1899, 1456, 2013,
1458, 2458, 1254, 1472, 2365,
1456, 2265, 1457, 2456};
System.out.println(contains(my_array1, 2013));
System.out.println(contains(my_array1, 2015));
}
}
Sample Output:
true false
Flowchart:
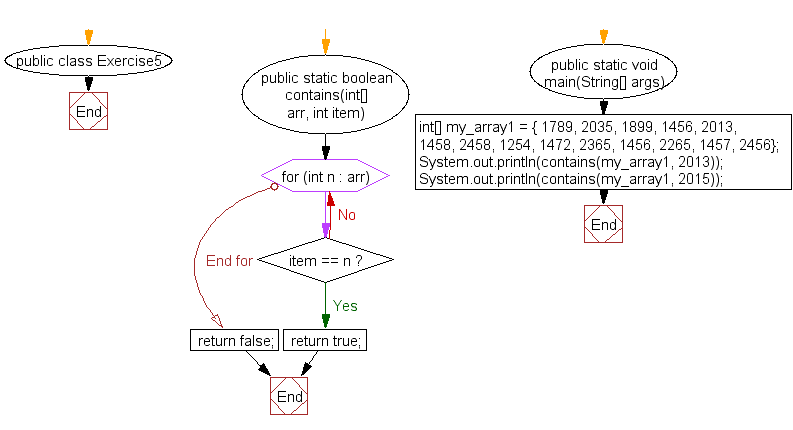
Visualize Java code execution (Python Tutor):
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to calculate the average value of array elements.
Next: Write a Java program to find the index of an array element.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework