Java Array Exercises: Find all combination of four elements of a given array whose sum is equal to a given value
Java Array: Exercise-43 with Solution
Write a Java program to find all combination of four elements of a given array whose sum is equal to a given value.
Pictorial Presentation:

Sample Solution:
Java Code:
import java.util.*;
import java.lang.*;
public class Main
{
public static void main (String[] args)
{
int nums[] = {10, 20, 30, 40, 1, 2};
int n = nums.length;
// given value
int s = 53;
System.out.println("Given value: "+s);
System.out.print("Combination of four elements:");
// Find other three elements after fixing first element
for (int i = 0; i < n - 3; i++)
{
// Find other two elements after fixing second element
for (int j = i + 1; j < n - 2; j++)
{
// Find the fourth element after fixing third element
for (int k = j + 1; k < n - 1; k++)
{
// find the fourth
for (int l = k + 1; l < n; l++)
{
if (nums[i] + nums[j] + nums[k] + nums[l] == s)
System.out.print("\n"+nums[i]+" "+nums[j]+" "+nums[k]
+" "+nums[l]);
}
}
}
}
}
}
Sample Output:
Given value: 53 Combination of four elements: 10 40 1 2 20 30 1 2
Flowchart:
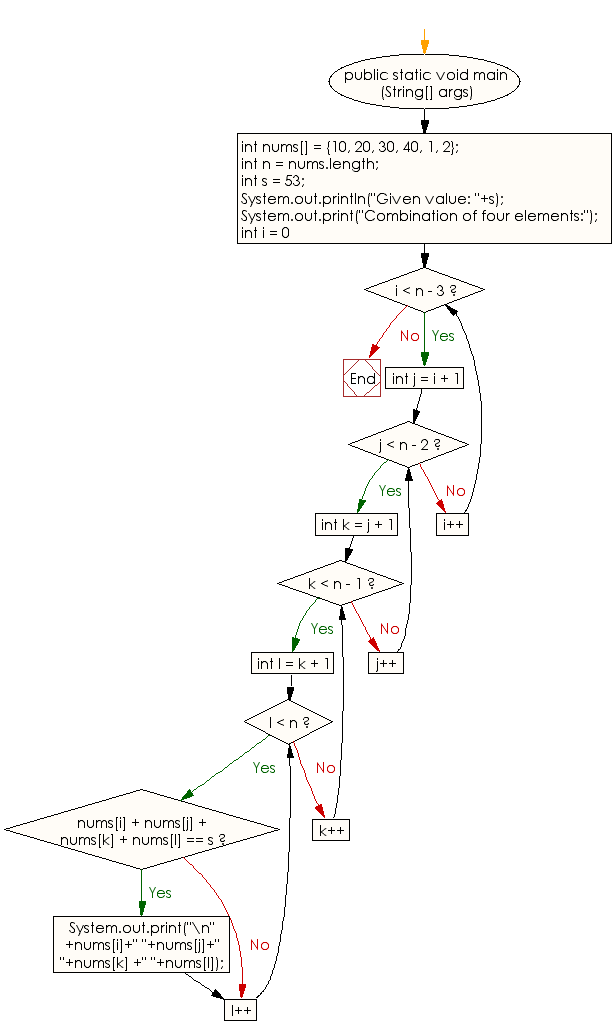
Visualize Java code execution (Python Tutor):
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to segregate all 0s on left side and all 1s on right side of a given array of 0s and 1s.
Next: Write a Java program to count the number of possible triangles from a given unsorted array of positive integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework