Java Array Exercises: Find the two elements from a given array of positive and negative numbers such that their sum is closest to zero
Java Array: Exercise-40 with Solution
Write a Java program to find the two elements from a given array of positive and negative numbers such that their sum is closest to zero.
Sample Solution:
Java Code:
import java.util.*;
import java.lang.*;
public class Main
{
public static void main (String[] args)
{
int arr[] = {1, 5, -4, 7, 8, -6};
int size = arr.length;
int l, r, min_sum, sum, min_l_num, min_r_num;
if(size < 2)
{
System.out.println("Invalid Input");
return;
}
min_l_num = 0;
min_r_num = 1;
min_sum = arr[0] + arr[1];
for(l = 0; l < size - 1; l++)
{
for(r = l+1; r < size; r++)
{
sum = arr[l] + arr[r];
if(Math.abs(min_sum) > Math.abs(sum))
{
min_sum = sum;
min_l_num = l;
min_r_num = r;
}
}
}
System.out.println("Two elements whose sum is minimum are "+
arr[min_l_num]+ " and "+arr[min_r_num]);
}
}
Sample Output:
Two elements whose sum is minimum are 5 and -4
Flowchart:
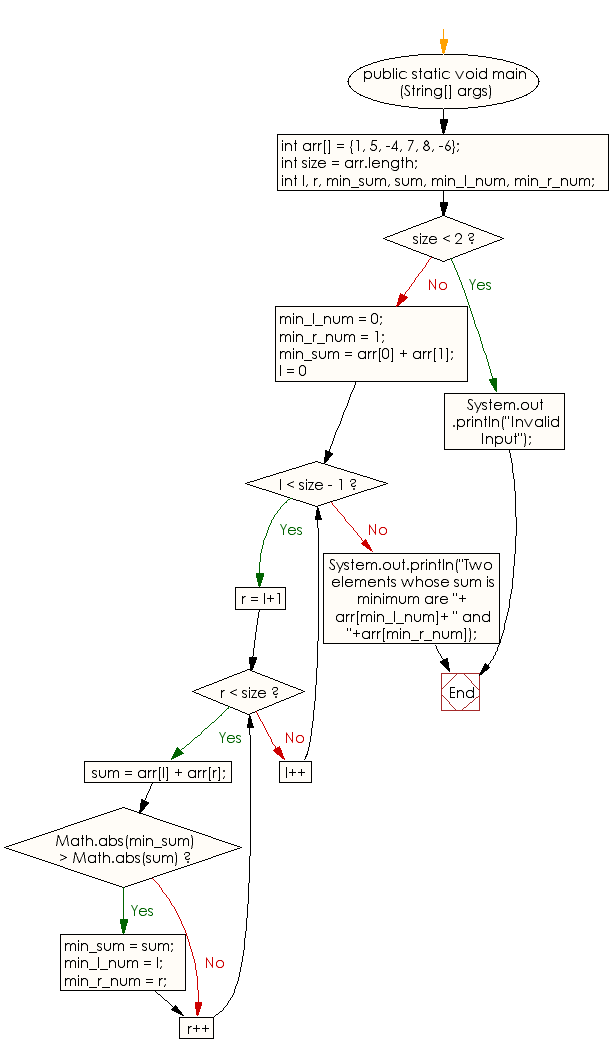
Visualize Java code execution (Python Tutor):
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to print all the LEADERS in the array.
Next: Write a Java program to find smallest and second smallest elements of a given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework