Java Array Exercises: Convert an array to ArrayList
Java Array: Exercise-20 with Solution
Write a Java program to convert an array to ArrayList.
Pictorial Presentation:
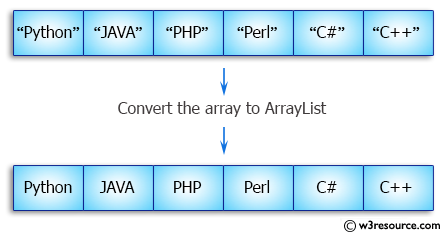
Sample Solution:
Java Code :
import java.util.ArrayList;
import java.util.Arrays;
public class Exercise20 {
public static void main(String[] args)
{
String[] my_array = new String[] {"Python", "JAVA", "PHP", "Perl", "C#", "C++"};
ArrayList<String> list = new ArrayList<String>(Arrays.asList(my_array));
System.out.println(list);
}
}
Sample Output:
[Python, JAVA, PHP, Perl, C#, C++]
Flowchart:
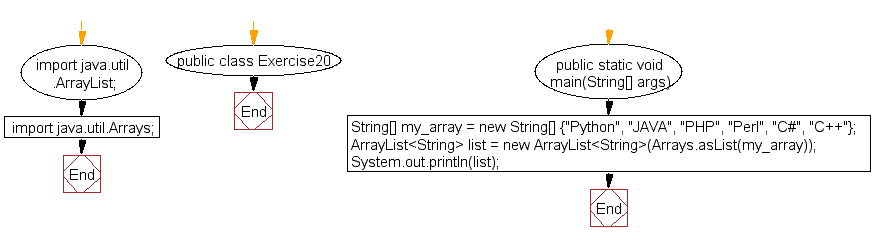
Visualize Java code execution (Python Tutor):
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to add two matrices of the same size.
Next: Write a Java program to convert an ArrayList to an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework