Java Array Exercises: Remove duplicate elements from an array
Java Array: Exercise-16 with Solution
Write a Java program to remove duplicate elements from an array.
Pictorial Presentation:
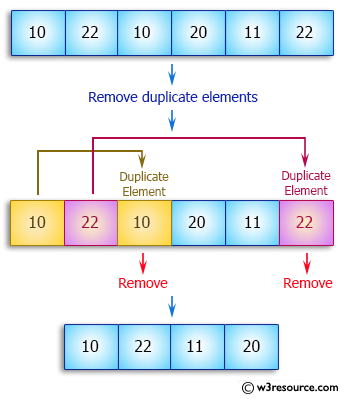
Sample Solution:
Java Code:
import java.util.Arrays;
public class Exercise16 {
static void unique_array(int[] my_array)
{
System.out.println("Original Array : ");
for (int i = 0; i < my_array.length; i++)
{
System.out.print(my_array[i]+"\t");
}
//Assuming all elements in input array are unique
int no_unique_elements = my_array.length;
//Comparing each element with all other elements
for (int i = 0; i < no_unique_elements; i++)
{
for (int j = i+1; j < no_unique_elements; j++)
{
//If any two elements are found equal
if(my_array[i] == my_array[j])
{
//Replace duplicate element with last unique element
my_array[j] = my_array[no_unique_elements-1];
no_unique_elements--;
j--;
}
}
}
//Copying only unique elements of my_array into array1
int[] array1 = Arrays.copyOf(my_array, no_unique_elements);
//Printing arrayWithoutDuplicates
System.out.println();
System.out.println("Array with unique values : ");
for (int i = 0; i < array1.length; i++)
{
System.out.print(array1[i]+"\t");
}
System.out.println();
System.out.println("---------------------------");
}
public static void main(String[] args)
{
unique_array(new int[] {0, 3, -2, 4, 3, 2});
unique_array(new int[] {10, 22, 10, 20, 11, 22});
}
}
Sample Output:
Original Array : 0 3 -2 4 3 2 Array with unique values : 0 3 -2 4 2 --------------------------- Original Array : 10 22 10 20 11 22 Array with unique values : 10 22 11 20 ---------------------------
Flowchart:
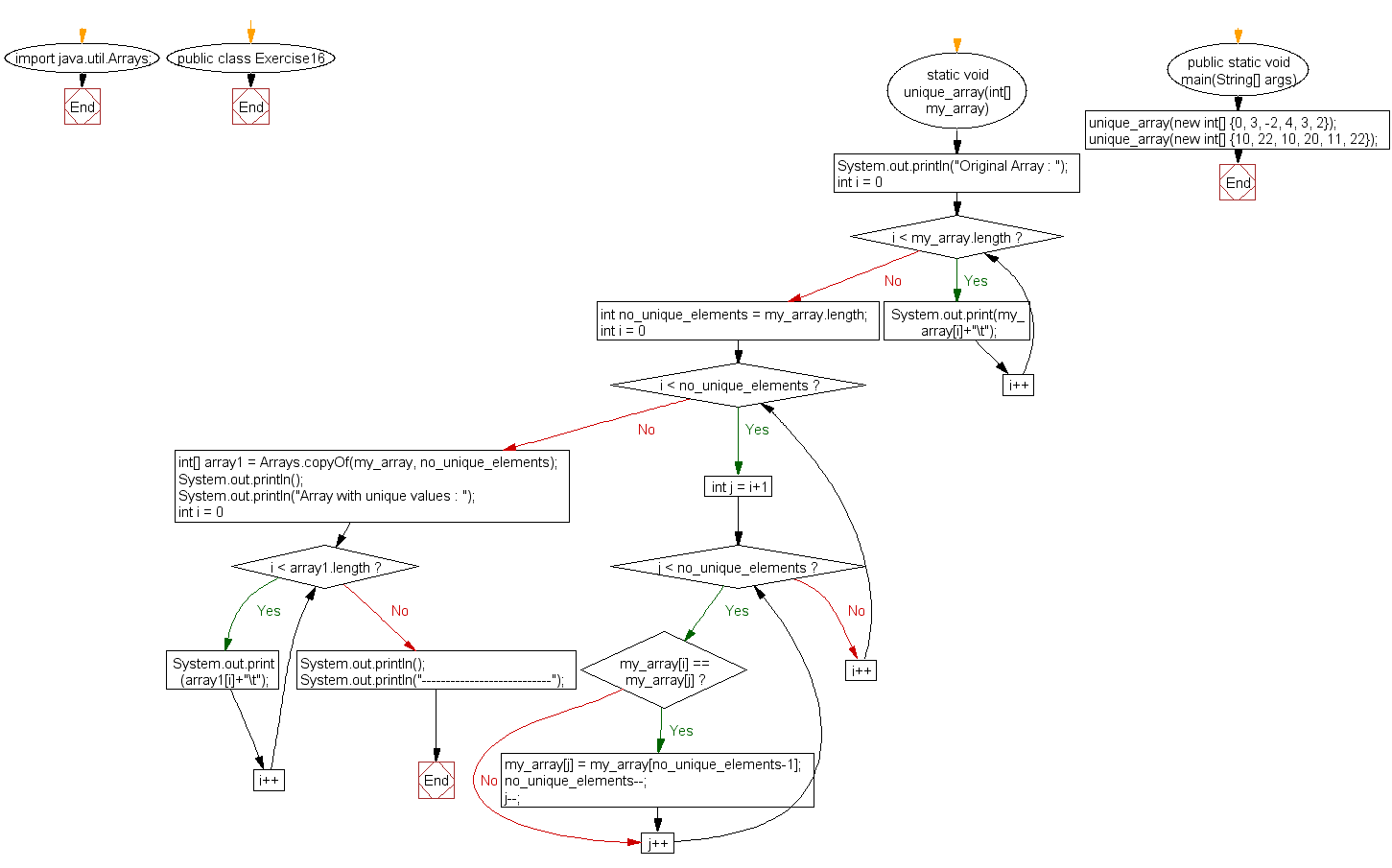
Visualize Java code execution (Python Tutor):
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to find the common elements between two arrays of integers.
Next: Write a Java program to find the second largest element in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework