C# Sharp Exercises: Structure initialization without using the new operator
C# Sharp STRUCTURE: Exercise-8 with Solution
Write a program in C# Sharp to demonstrates structure initialization without using the new operator.
Sample Solution:-
C# Sharp Code:
using System;
public struct newStruct
{
public int m, n;
public newStruct(int pt1, int pt2)
{
m = pt1;
n = pt2;
}
}
// Declare and initialize struct objects.
class strucExer8
{
static void Main()
{
Console.Write("\n\nStructure initialization without using the new operator :\n");
Console.Write("----------------------------------------------------------\n");
newStruct myPoint;
myPoint.m = 30;
myPoint.n = 40;
Console.Write("\nnewStruct : ");
Console.WriteLine("m = {0}, n = {1}", myPoint.m, myPoint.n);
Console.WriteLine("\nPress any key to exit.");
Console.ReadKey();
}
}
Sample Output:
Structure initialization without using the new operator : ---------------------------------------------------------- newStruct : m = 30, n = 40 Press any key to exit.
Flowchart:
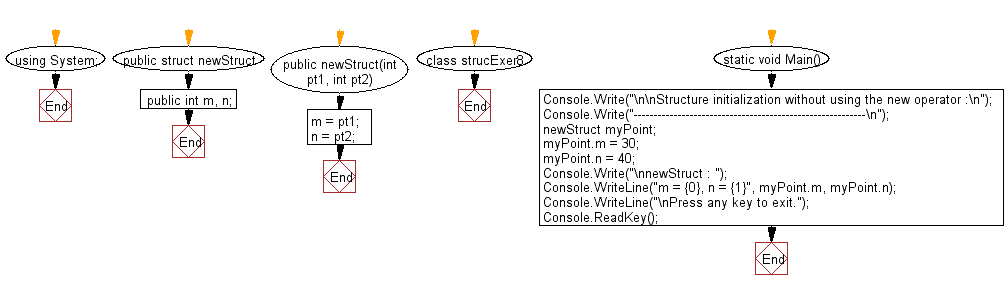
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to demonstrates structure initialization using both default and parameterized constructors.
Next: Write a program in C# Sharp to insert the information of two books.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework