C# Sharp Exercises: Creates two string-objects with different value
C# Sharp String: Exercise-39 with Solution
Write a C# Sharp program to create two string-objects with different values. When it calls the Copy method to assign the first value to the second string, the output indicates that the strings represent different object references although their values are now equal. On the other hand, when the first string is assigned to the second string, the two strings have identical values because they represent the same object reference.
Sample Solution:-
C# Sharp Code:
using System;
class Example39
{
public static void Main()
{
string s1 = "JAVA";
string s2 = "Python";
Console.WriteLine("s1 = '{0}'", s1);
Console.WriteLine("s2 = '{0}'", s2);
Console.WriteLine("\nAfter String.Copy...");
s2 = String.Copy(s1);
Console.WriteLine("s1 = '{0}'", s1);
Console.WriteLine("s2 = '{0}'", s2);
Console.WriteLine("ReferenceEquals: {0}", Object.ReferenceEquals(s1, s2));
Console.WriteLine("Equals: {0}", Object.Equals(s1, s2));
Console.WriteLine("\nAfter Assignment...");
s2 = s1;
Console.WriteLine("s1 = '{0}'", s1);
Console.WriteLine("s2 = '{0}'", s2);
Console.WriteLine("ReferenceEquals: {0}", Object.ReferenceEquals(s1, s2));
Console.WriteLine("Equals: {0}", Object.Equals(s1, s2));
}
}
Sample Output:
s1 = 'JAVA' s2 = 'Python' After String.Copy... s1 = 'JAVA' s2 = 'JAVA' ReferenceEquals: False Equals: True After Assignment... s1 = 'JAVA' s2 = 'JAVA' ReferenceEquals: True Equals: True
Flowchart :
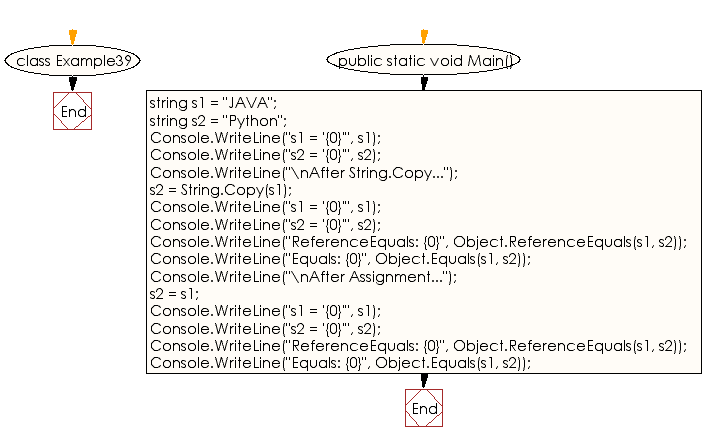
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to determine whether the string "birds" is a substring of a familiar.
Next: Write a C# Sharp program to demonstrates the CopyTo method.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework