C# Sharp Exercises: Compare two substrings using different cultures and ignoring the case of the substrings
C# Sharp String: Exercise-23 with Solution
Write a C# Sharp program to compare two substrings using different cultures and ignoring the case of the substrings.
Sample Solution:-
C# Sharp Code:
// Example for String.Compare(String, Int32, String, Int32, Int32, Boolean, CultureInfo)
using System;
using System.Globalization;
class Example23 {
public static void Main() {
// 01234567
String str1 = "COMPUTER";
String str2 = "computer";
String str;
int result;
Console.WriteLine();
Console.WriteLine("\n str1 = '{0}', str2 = '{1}'", str1, str2);
Console.WriteLine("Ignore case, Turkish culture:");
result = String.Compare(str1, 4, str2, 4, 2, true, new CultureInfo("tr-TR"));
str = ((result < 0) ? "less than" : ((result > 0) ? "greater than" : "equal to"));
Console.Write("Substring '{0}' in '{1}' is ", str1.Substring(4, 2), str1);
Console.Write("{0} ", str);
Console.WriteLine("substring '{0}' in '{1}'.", str2.Substring(4, 2), str2);
Console.WriteLine();
Console.WriteLine("Ignore case, invariant culture:");
result = String.Compare(str1, 4, str2, 4, 2, true, CultureInfo.InvariantCulture);
str = ((result < 0) ? "less than" : ((result > 0) ? "greater than" : "equal to"));
Console.Write("Substring '{0}' in '{1}' is ", str1.Substring(4, 2), str1);
Console.Write("{0} ", str);
Console.WriteLine("substring '{0}' in '{1}'.\n\n", str2.Substring(4, 2), str2);
}
}
Sample Output:
str1 = 'COMPUTER', str2 = 'computer' Ignore case, Turkish culture: Substring 'UT' in 'COMPUTER' is equal to substring 'ut' in 'computer'. Ignore case, invariant culture: Substring 'UT' in 'COMPUTER' is equal to substring 'ut' in 'computer'.
Flowchart :
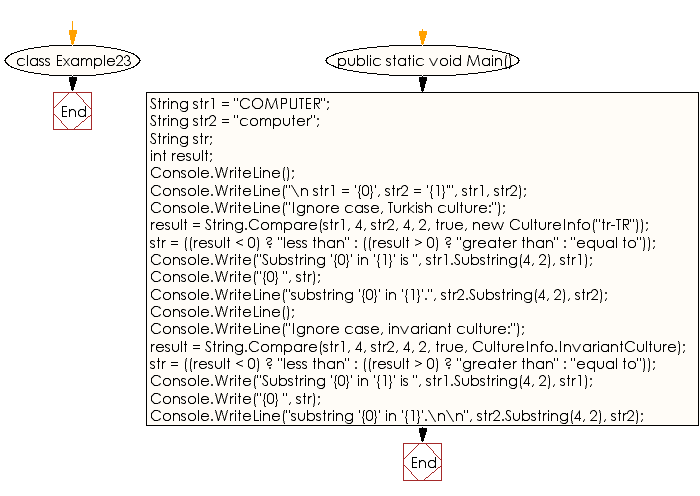
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compare two substrings that only differ in case. The first comparison ignores case and the second comparison considers case.
Next: Write a C# Sharp program to compare the last names of two people. It then lists them in alphabetical order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework