C# Sharp Exercises: Replace lowercase characters by uppercase and vice-versa
C# Sharp String: Exercise-15 with Solution
Write a program in C# Sharp to read a sentence and replace lowercase characters by uppercase and vice-versa.
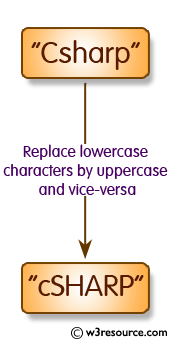
Sample Solution:-
C# Sharp Code:
using System;
public class exercise15
{
public static void Main()
{
string str1;
char[] arr1;
int l,i;
l=0;
char ch;
Console.Write("\n\nReplace lowercase characters by uppercase and vice-versa :\n");
Console.Write("--------------------------------------------------------------\n");
Console.Write("Input the string : ");
str1 = Console.ReadLine();
l=str1.Length;
arr1 = str1.ToCharArray(0, l); // Converts string into char array.
Console.Write("\nAfter conversion, the string is : ");
for(i=0; i < l; i++)
{
ch=arr1[i];
if (Char.IsLower(ch)) // check whether the character is lowercase
Console.Write(Char.ToUpper(ch)); // Converts lowercase character to uppercase.
else
Console.Write(Char.ToLower(ch)); // Converts uppercase character to lowercase.
}
Console.Write("\n\n");
}
}
Sample Output:
Replace lowercase characters by uppercase and vice-versa : -------------------------------------------------------------- Input the string : w3RESOURCE After conversion, the string is : W3resource
Flowchart:
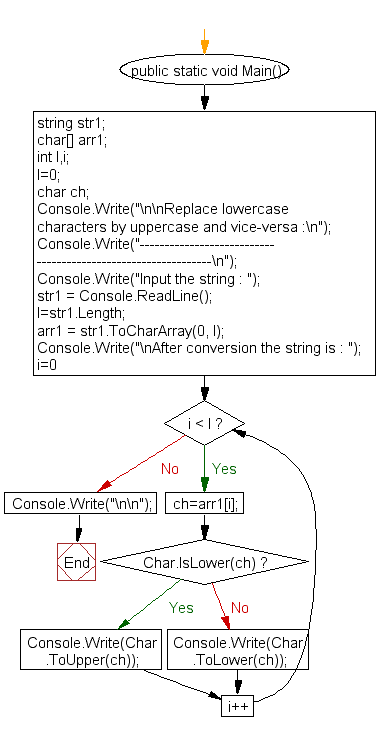
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to check whether a given substring is present in the given string
Next: Write a program in C# Sharp to check the username and password.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework