C# Sharp Exercises: Sorts the strings of an array using bubble sort
C# Sharp String: Exercise-12 with Solution
Write a program in C# Sharp to read a string through the keyboard and sort it using bubble sort.
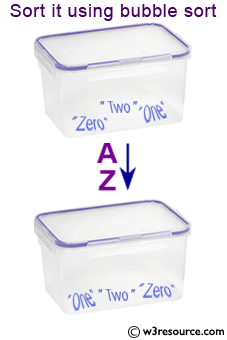
Sample Solution:-
C# Sharp Code:
using System;
public class exercise12
{
public static void Main()
{
string[] arr1;
string temp;
int n,i,j,l;
Console.Write("\n\nSorts the strings of an array using bubble sort :\n");
Console.Write("-----------------------------------------------------\n");
Console.Write("Input number of strings :");
n= Convert.ToInt32(Console.ReadLine());
arr1=new string[n];
Console.Write("Input {0} strings below :\n",n);
for(i=0;i<n;i++)
{
arr1[i] = Console.ReadLine();
}
l=arr1.Length;
for (i = 0; i < l; i++)
{
for (j = 0; j < l-1; j++)
{
if (arr1[j].CompareTo(arr1[j + 1]) > 0)
{
temp = arr1[j];
arr1[j] = arr1[j + 1];
arr1[j + 1] = temp;
}
}
}
Console.Write("\n\nAfter sorting the array appears like : \n");
for (i = 0; i < l; i++)
{
Console.WriteLine(arr1[i] + " ");
}
}
}
Sample Output:
Sorts the strings of an array using bubble sort : ----------------------------------------------------- Input number of strings :3 Input 3 strings below : IJKL EFGH ABCD After sorting the array appears like : ABCD EFGH IJKL
Flowchart:
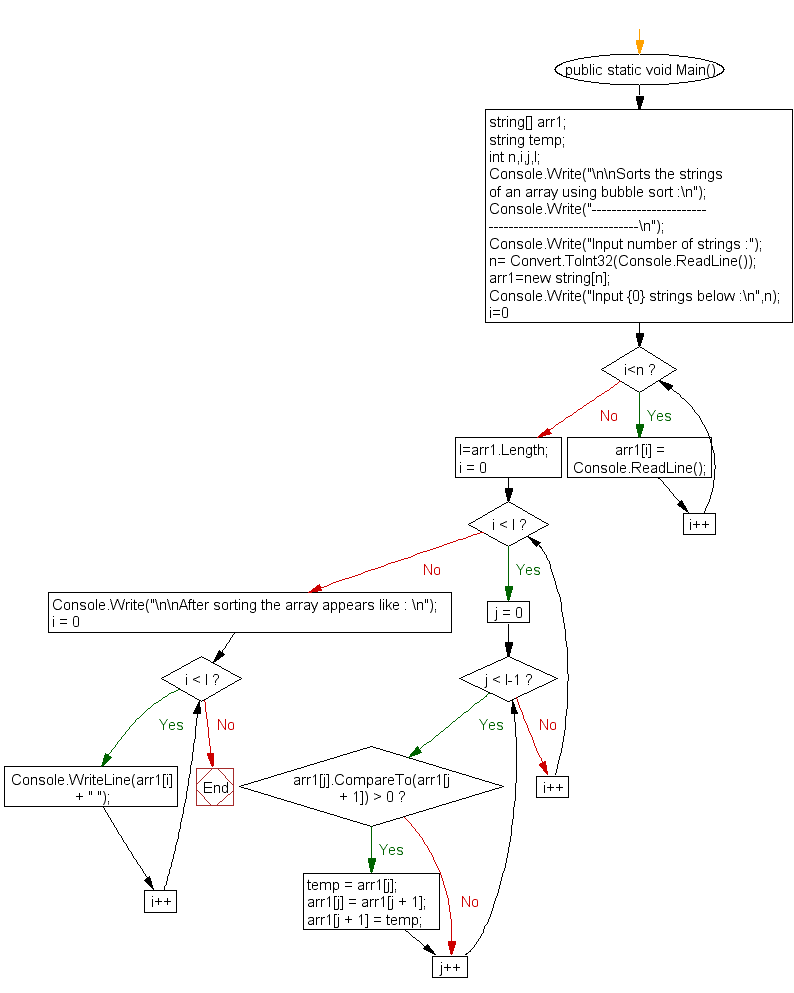
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to sort a string array in ascending order.
Next: Write a program in C# Sharp to extract a substring from a given string without using the library function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework