C# Sharp Exercises: Sum of first n natural numbers
C# Sharp Recursion : Exercise-3 with Solution
Write a program in C# Sharp to find the sum of first n natural numbers using recursion.
Pictorial Presentation:
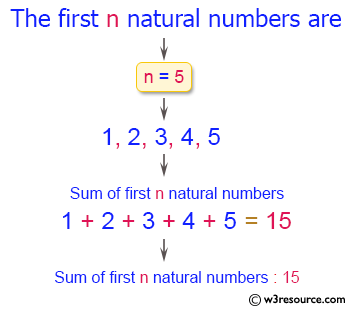
Sample Solution:
C# Sharp Code:
using System;
class RecExercise3
{
static void Main(string[] args)
{
Console.Write("\n\n Recursion : Sum of first n natural numbers :\n");
Console.Write("--------------------------------------------------\n");
Console.Write(" How many numbers to sum : ");
int n = Convert.ToInt32(Console.ReadLine());
Console.Write(" The sum of first {0} natural numbers is : {1}\n\n", n,SumOfTen(1,n));
}
static int SumOfTen(int min, int max)
{
return CalcuSum(min, max);
}
static int CalcuSum(int min, int val)
{
if (val == min)
return val;
return val + CalcuSum(min, val - 1);
}
}
Sample Output:
Recursion : Sum of first n natural numbers : -------------------------------------------------- How many numbers to sum : 5 The sum of first 5 natural numbers is : 15
Flowchart :
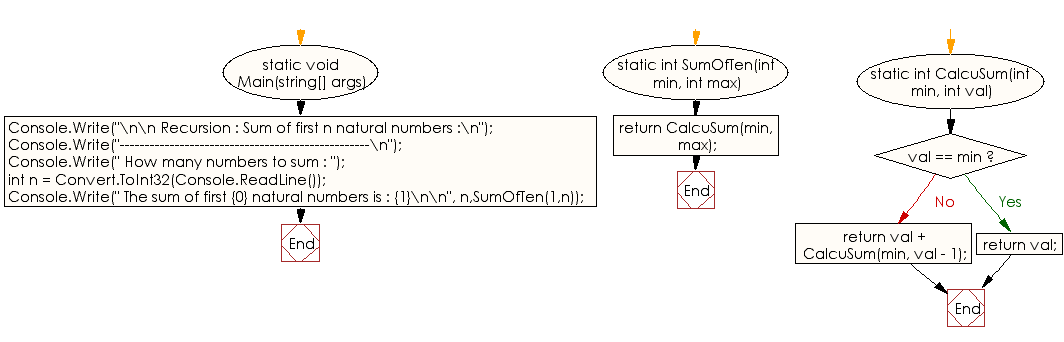
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to print numbers from n to 1 using recursion.
Next: Write a program in C# Sharp to display the individual digits of a given number using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework