C# Sharp Exercises: Get the reverse of a string
C# Sharp Recursion: Exercise-14 with Solution
Write a program in C# Sharp to get the reverse of a string using recursion.
Pictorial Presentation:
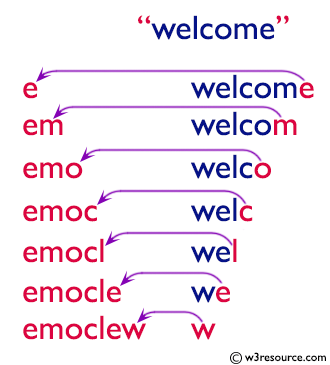
Sample Solution:
C# Sharp Code:
using System;
class RecExercise14
{
static void Main()
{
string str;
Console.WriteLine("\n\n Recursion : Get the reverse of a string :");
Console.WriteLine("----------------------------------------------");
Console.Write(" Input the string : ");
str = Console.ReadLine();
str = StringReverse(str);
Console.Write(" The reverse of the string is : ");
Console.Write(str);
Console.ReadKey();
Console.Write("\n");
}
public static string StringReverse(string str)
{
if (str.Length > 0)
return str[str.Length - 1] + StringReverse(str.Substring(0, str.Length - 1));
else
return str;
}
}
Sample Output:
Recursion : Get the reverse of a string : ---------------------------------------------- Input the string : W3resource The reverse of the string is : ecruoser3W
Flowchart :

C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to convert a decimal number to binary using recursion.
Next: Write a program in C# Sharp to calculate the power of any number using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework