C# Sharp Exercises: Calculate true mean value, mean with rounding away from zero and mean with rounding to nearest
C# Sharp Math: Exercise-4 with Solution
Write a C# Sharp program to calculate true mean value, mean with rounding away from zero and mean with rounding to nearest of some specified decimal values.
Sample Solution:
C# Sharp Code:
using System;
using System.Text;
namespace exercises {
class Program {
static void Main(string[] args) {
decimal[] values = {
10.23m,
10.27m,
14.34m,
11.15m,
41.51m,
10.65m
};
decimal sum = 0;
// Calculate true mean.
foreach(var value in values)
sum += value;
Console.WriteLine("True Mean: {0:N2}", sum / values.Length);
// Calculate mean with rounding away from zero.
sum = 0;
foreach(var value in values)
sum += Math.Round(value, 1, MidpointRounding.AwayFromZero);
Console.WriteLine("Away From Zero: {0:N2}", sum / values.Length);
// Calculate mean with rounding to nearest.
sum = 0;
foreach(var value in values)
sum += Math.Round(value, 1, MidpointRounding.ToEven);
Console.WriteLine("Rounding to Nearest: {0:N2}", sum / values.Length);
}
}
}
Sample Output:
True Mean: 16.36 Away From Zero: 16.37 Rounding to Nearest: 16.35
Flowchart:
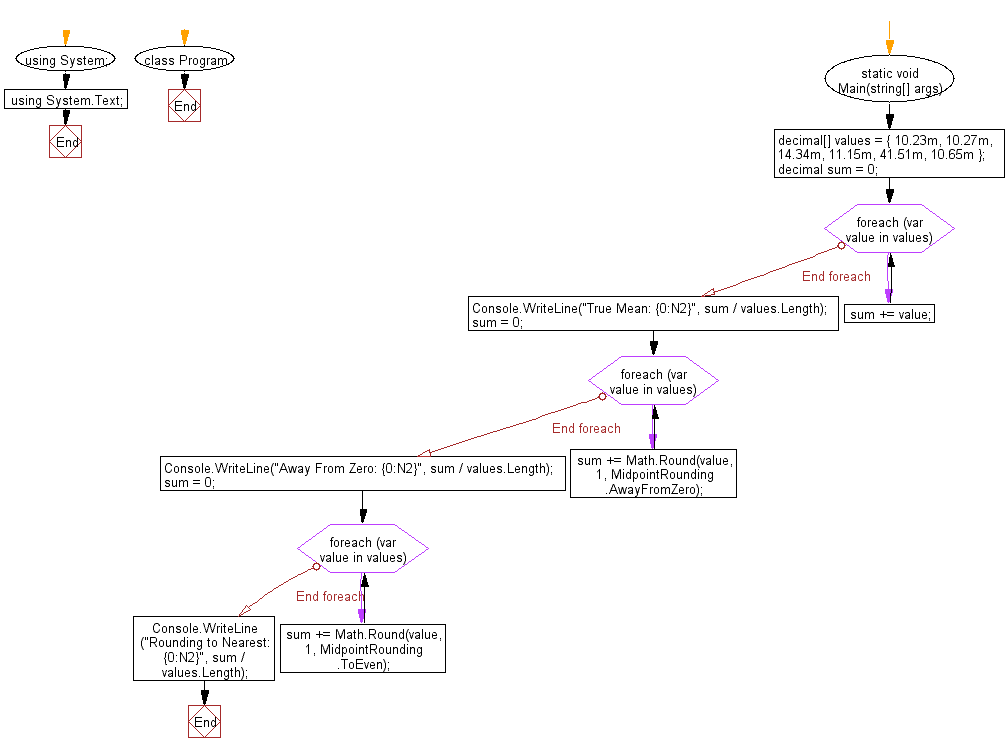
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to calculate the value that results from raising 3 to a power ranging from 0 to 32.
Next: Write a C# Sharp program to determine the sign of a single value and display it to the console.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework