C# Sharp Exercises: Convert a given string value to a 32-bit signed integer
C# Sharp Math: Exercise-11 with Solution
Write a C# Sharp program to convert a given string value to a 32-bit signed integer.
Sample Solution:
C# Sharp Code:
using System;
namespace exercises {
class Program {
static void Main(string[] args) {
String str1;
str1 = "123456";
Console.WriteLine("Original String value: " + str1);
Console.WriteLine("Convert the said string to integer value:");
Console.WriteLine(str_to_integer(str1));
str1 = "+3456";
Console.WriteLine("Original String value: " + str1);
Console.WriteLine("Convert the said string to integer value:");
Console.WriteLine(str_to_integer(str1));
str1 = "-123456";
Console.WriteLine("Original String value: " + str1);
Console.WriteLine("Convert the said string to integer value:");
Console.WriteLine(str_to_integer(str1));
str1 = "a1234";
Console.WriteLine("Original String value: " + str1);
Console.WriteLine("Convert the said string to integer value:");
Console.WriteLine(str_to_integer(str1));
str1 = "123a456";
Console.WriteLine("Original String value: " + str1);
Console.WriteLine("Convert the said string to integer value:");
Console.WriteLine(str_to_integer(str1));
}
public static int str_to_integer(String str1)
{
if (string.IsNullOrWhiteSpace(str1)) { return 0; }
var flag = false;
var index_num = 0;
while (index_num < str1.Length && str1[index_num] == ' ') { index_num++; }
if (str1[index_num] == '-')
{
flag = true;
index_num++;
}
else if (str1[index_num] == '+')
{
index_num++;
}
var max1 = int.MaxValue / 10;
var max2 = int.MaxValue % 10;
var result = 0;
for (; index_num < str1.Length; index_num++)
{
var str_digit = str1[index_num] - '0';
if (str_digit < 0 || str_digit > 9) { break; }
if (result > max1 ||
(result == max1 && str_digit > max2))
{
return flag ? int.MinValue : int.MaxValue;
}
result = result * 10 + str_digit;
}
return flag ? -result : result;
}
}
}
Sample Output:
Original String value: 123456 Convert the said string to integer value: 123456 Original String value: +3456 Convert the said string to integer value: 3456 Original String value: -123456 Convert the said string to integer value: -123456 Original String value: a1234 Convert the said string to integer value: 0 Original String value: 123a456 Convert the said string to integer value: 123
Flowchart:
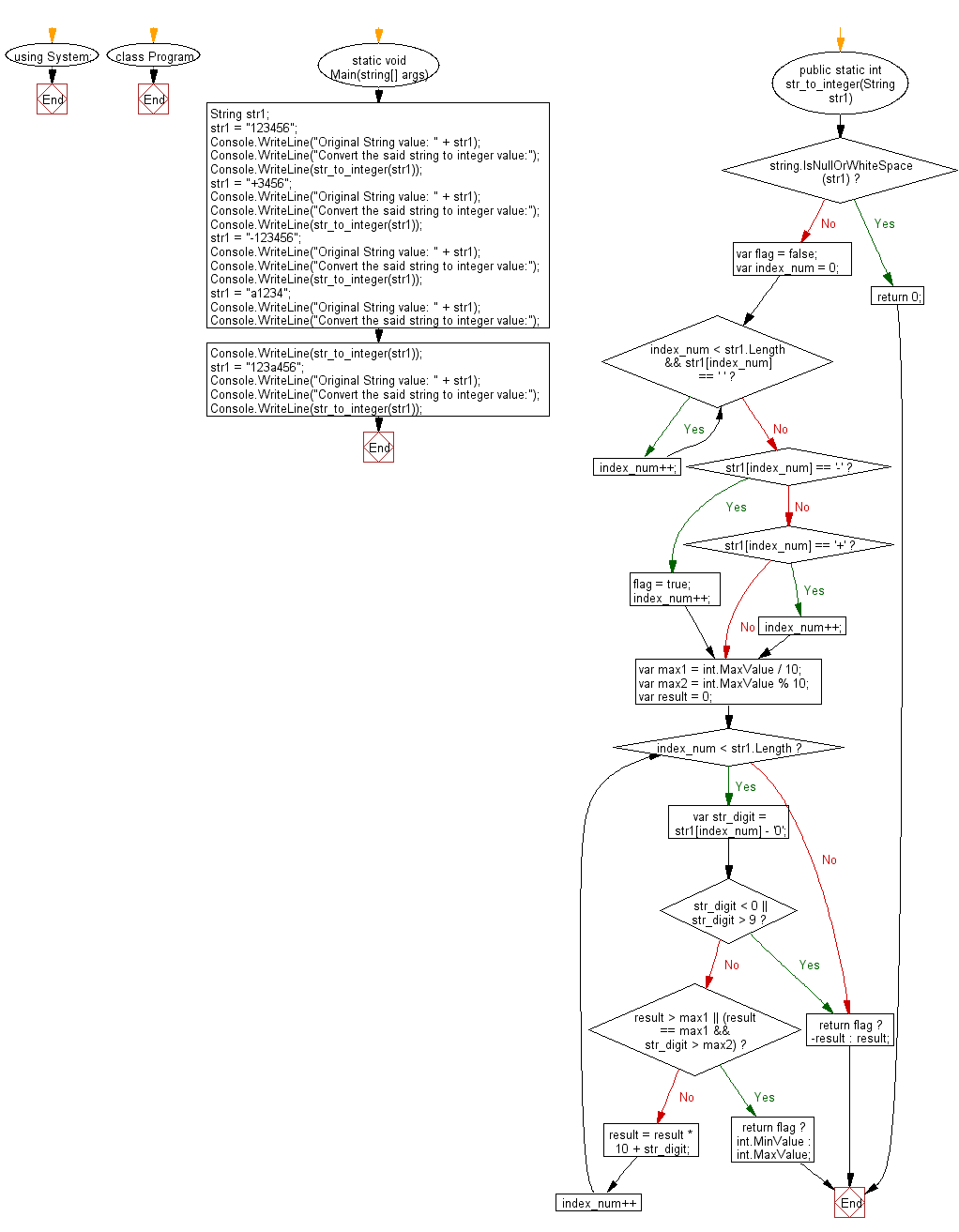
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to reverse the digits of a given signed 32-bit integer.
Next: Write a C# Sharp program to check whether a given integer is a palindrome integer or not. Return true if the number is palindrome otherwise return false.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework