C# Sharp Exercises: Split a collection of strings into some groups
C# Sharp LINQ : Exercise-29 with Solution
Write a program in C# Sharp to split a collection of strings into some groups.
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
class LinqExercise29
{
static void Main(string[] args)
{
string[] cities =
{
"ROME","LONDON","NAIROBI","CALIFORNIA",
"ZURICH","NEW DELHI","AMSTERDAM",
"ABU DHABI", "PARIS","NEW YORK"
};
Console.Write("\nLINQ : Split a collection of strings into some groups : ");
Console.Write("\n-------------------------------------------------------\n");
Console.Write("\nThe cities are : 'ROME','LONDON','NAIROBI','CALIFORNIA','ZURICH','NEW DELHI', \n");
Console.Write(" 'AMSTERDAM','ABU DHABI','PARIS','NEW YORK' \n");
Console.Write("\nHere is the group of cities : \n\n");
var citySplit = from i in Enumerable.Range(0, cities.Length)
group cities[i] by i / 3;
foreach(var city in citySplit)
cityView(string.Join("; ", city.ToArray()));
Console.ReadLine();
}
static void cityView(string cityMetro)
{
Console.WriteLine(cityMetro);
Console.WriteLine("-- here is a group of cities --\n");
}
}
Sample Output:
LINQ : Split a collection of strings into some groups : ------------------------------------------------------- The cities are : 'ROME','LONDON','NAIROBI','CALIFORNIA','ZURICH','NEW DELHI', 'AMSTERDAM','ABU DHABI','PARIS','NEW YORK' Here is the group of cities : ROME; LONDON; NAIROBI -- here is a group of cities -- CALIFORNIA; ZURICH; NEW DELHI -- here is a group of cities -- AMSTERDAM; ABU DHABI; PARIS -- here is a group of cities -- NEW YORK -- here is a group of cities --
Pictorial Presentation:
Flowchart:
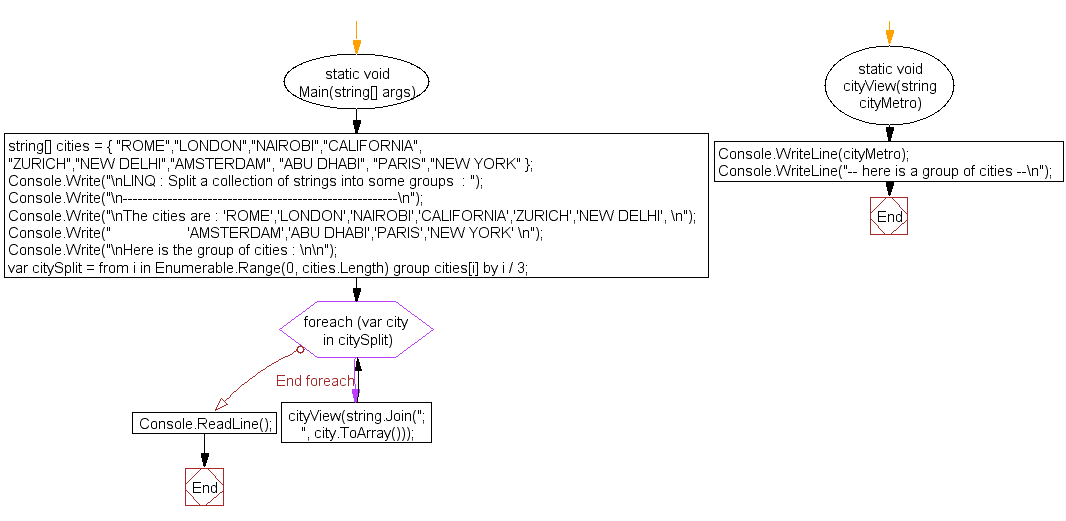
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the list of items in the array according to the length of the string then by name in ascending order.
Next: Write a program in C# Sharp to arrange the distinct elements in the list in ascending order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework