C# Sharp Exercises: Function : To swap the values of two integer numbers
C# Sharp Function: Exercise-6 with Solution
Write a program in C# Sharp to create a function to swap the values of two integer numbers.
Pictorial Presentation:
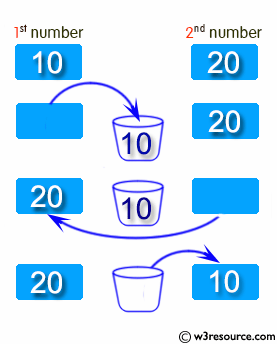
Sample Solution:
C# Sharp Code:
using System;
public class funcexer6
{
public static void interchange(ref int num1, ref int num2)
{
int newnum;
newnum = num1;
num1 = num2;
num2 = newnum;
}
public static void Main()
{
int n1,n2;
Console.Write("\n\nFunction : To swap the values of two integer numbers :\n");
Console.Write("----------------------------------------------------------\n");
Console.Write("Enter a number: ");
n1= Convert.ToInt32(Console.ReadLine());
Console.Write("Enter another number: ");
n2= Convert.ToInt32(Console.ReadLine());
interchange( ref n1, ref n2 );
Console.WriteLine( "Now the 1st number is : {0} , and the 2nd number is : {1}", n1, n2 );
}
}
Sample Output:
Function : To swap the values of two integer numbers : ---------------------------------------------------------- Enter a number: 45 Enter another number: 65 Now the 1st number is : 65 , and the 2nd number is : 45
Flowchart :
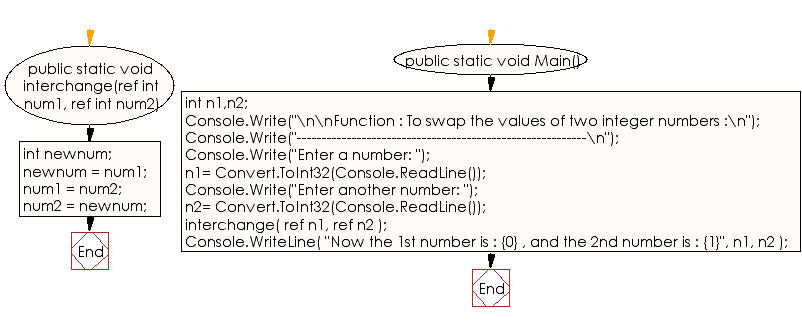
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to calculate the sum of elements in an array.
Next: Write a program in C# Sharp to create a function to calculate the result of raising an integer number to another.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework