C# Sharp Exercises: Determine the LCM of two numbers using HCF
C# Sharp For Loop: Exercise-44 with Solution
Write a program in C# Sharp to find LCM of any two numbers using HCF.
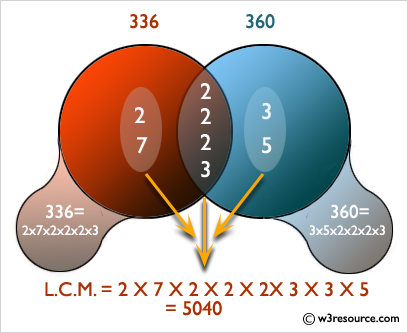
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise44
{
public static void Main()
{
int i, n1, n2, j, hcf=1,lcm;
Console.Write("\n\n");
Console.Write("Determine the LCM of two numbers using HCF:\n");
Console.Write("---------------------------------------------");
Console.Write("\n\n");
Console.Write("Input 1st number for LCM: ");
n1 = Convert.ToInt32(Console.ReadLine());
Console.Write("Input 2nd number for LCM: ");
n2 = Convert.ToInt32(Console.ReadLine());
j = (n1<n2) ? n1 : n2;
for(i=1; i<=j; i++)
{
if(n1%i==0 && n2%i==0)
{
hcf = i;
}
}
/* We know the multiplication of HCF and LCM is equivalant
to the multiplication of these two numbers.*/
lcm=(n1*n2)/hcf;
Console.Write("\nThe LCM of {0} and {1} is : {2}\n\n", n1, n2, lcm);
}
}
Sample Output:
Determine the LCM of two numbers using HCF: --------------------------------------------- Input 1st number for LCM: 8 Input 2nd number for LCM: 12 The LCM of 8 and 12 is : 24
Flowchart:
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to find HCF (Highest Common Factor) of two numbers.
Next: Write a program in C# Sharp to find LCM of any two numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework