C# Sharp Exercises: A menu-driven program for a simple calculator
C# Sharp Conditional Statement: Exercise-25 with Solution
Write a program in C# Sharp which is a Menu-Driven Program to perform a simple calculation.
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise25
{
public static void Main()
{
int num1,num2,opt;
Console.Write("\n\n");
Console.Write("A menu driven program for a simple calculator:\n");
Console.Write("------------------------------------------------");
Console.Write("\n\n");
Console.Write("Enter the first Integer :");
num1 = Convert.ToInt32(Console.ReadLine());
Console.Write("Enter the second Integer :");
num2 = Convert.ToInt32(Console.ReadLine());
Console.Write("\nHere are the options :\n");
Console.Write("1-Addition.\n2-Substraction.\n3-Multiplication.\n4-Division.\n5-Exit.\n");
Console.Write("\nInput your choice :");
opt = Convert.ToInt32(Console.ReadLine());
switch(opt) {
case 1:
Console.Write("The Addition of {0} and {1} is: {2}\n",num1,num2,num1+num2);
break;
case 2:
Console.Write("The Substraction of {0} and {1} is: {2}\n",num1,num2,num1-num2);
break;
case 3:
Console.Write("The Multiplication of {0} and {1} is: {2}\n",num1,num2,num1*num2);
break;
case 4:
if(num2==0) {
Console.Write("The second integer is zero. Devide by zero.\n");
} else {
Console.Write("The Division of {0} and {1} is : {2}\n",num1,num2,num1/num2);
}
break;
case 5:
break;
default:
Console.Write("Input correct option\n");
break;
}
}
}
Sample Output:
A menu driven program for a simple calculator: ------------------------------------------------ Enter the first Integer :20 Enter the second Integer :4 Here are the options : 1-Addition. 2-Substraction. 3-Multiplication. 4-Division. 5-Exit. Input your choice :4 The Division of 20 and 4 is : 5
Flowchart:
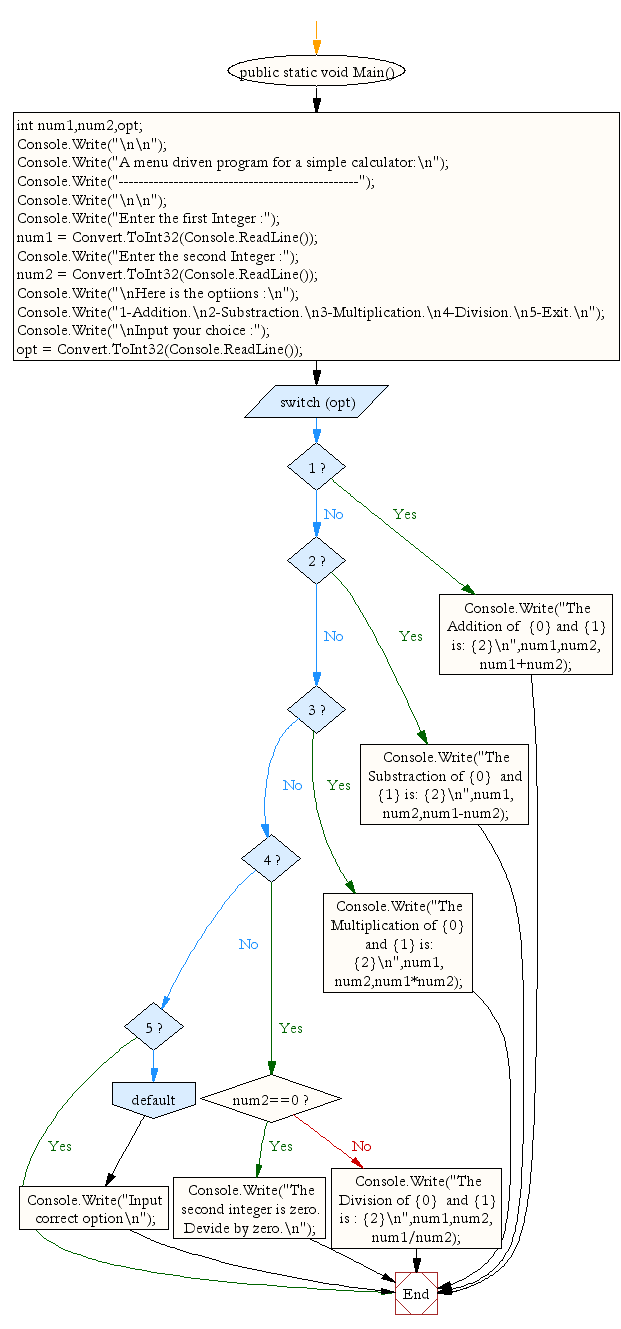
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp which is a Menu-Driven Program to compute the area of the various geometrical shape.
Next: C# Sharp programming exercises: For Loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework