C# Sharp Exercises: Check whether an alphabet is a vowel or consonant
C# Sharp Conditional Statement: Exercise-16 with Solution
Write a C# Sharp program to check whether an alphabet is a vowel or consonant.
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
public class exercise16
{
static void Main(string[] args)
{
char ch;
Console.Write("\n\n");
Console.Write("check whether the input alphabet is a vowel or not:\n");
Console.Write("-----------------------------------------------------");
Console.Write("\n\n");
Console.Write("Input an Alphabet (A-Z or a-z) : ");
ch = Convert.ToChar(Console.ReadLine().ToLower());
int i=ch;
if(i>=48 && i<=57)
{
Console.Write("You entered a number, Please enter an alpahbet.");
}
else
{
switch (ch)
{
case 'a':
Console.WriteLine("The Alphabet is vowel");
break;
case 'i':
Console.WriteLine("The Alphabet is vowel");
break;
case 'o':
Console.WriteLine("The Alphabet is vowel");
break;
case 'u':
Console.WriteLine("The Alphabet is vowel");
break;
case 'e':
Console.WriteLine("The Alphabet is vowel");
break;
default:
Console.WriteLine("The Alphabet is not a vowel");
break;
}
}
Console.ReadKey();
}
}
Sample Output:
check whether the input alphabet is a vowel or not: ----------------------------------------------------- Input an Alphabet : A The Alphabet is vowel
Pictorial Presentation:
Flowchart:
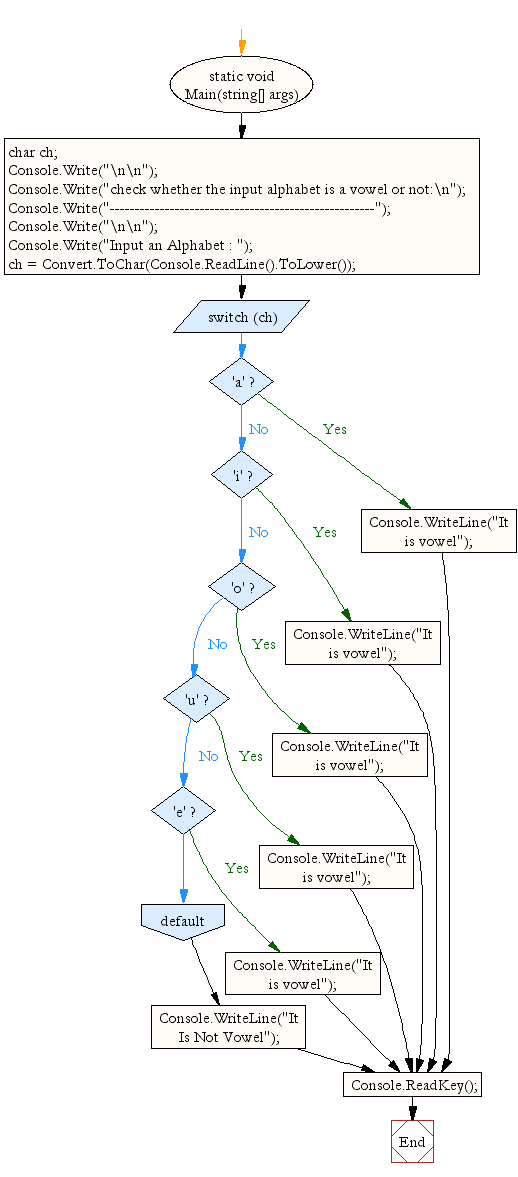
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to check whether a triangle can be formed by the given value for the angles.
Next: Write a C# Sharp program to calculate profit and loss on a transaction.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework