C# Sharp Exercises: Check whether a triangle is Equilateral, Isosceles or Scalene
C# Sharp Conditional Statement: Exercise-14 with Solution
Write a C# Sharp program to check whether a triangle is Equilateral, Isosceles or Scalene.
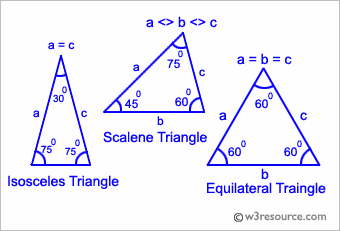
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise14
{
public static void Main()
{
int sidea, sideb, sidec;
Console.Write("\n\n");
Console.Write("Check whether a triangle is Equilateral, Isosceles or Scalene:\n");
Console.Write("----------------------------------------------------------------");
Console.Write("\n\n");
Console.Write("Input side 1 of triangle: ");
sidea= Convert.ToInt32(Console.ReadLine());
Console.Write("Input side 2 of triangle: ");
sideb= Convert.ToInt32(Console.ReadLine());
Console.Write("Input side 3 of triangle: ");
sidec= Convert.ToInt32(Console.ReadLine());
if(sidea==sideb && sideb==sidec)
{
Console.Write("This is an equilateral triangle.\n");
}
else if(sidea==sideb || sidea==sidec || sideb==sidec)
{
Console.Write("This is an isosceles triangle.\n");
}
else
{
Console.Write("This is a scalene triangle.\n");
}
}
}
Sample Output:
Check whether a triangle is Equilateral, Isosceles or Scalene: ---------------------------------------------------------------- Input side 1 of triangle: 40 Input side 2 of triangle: 20 Input side 3 of triangle: 20 This is an isosceles triangle.
Flowchart:
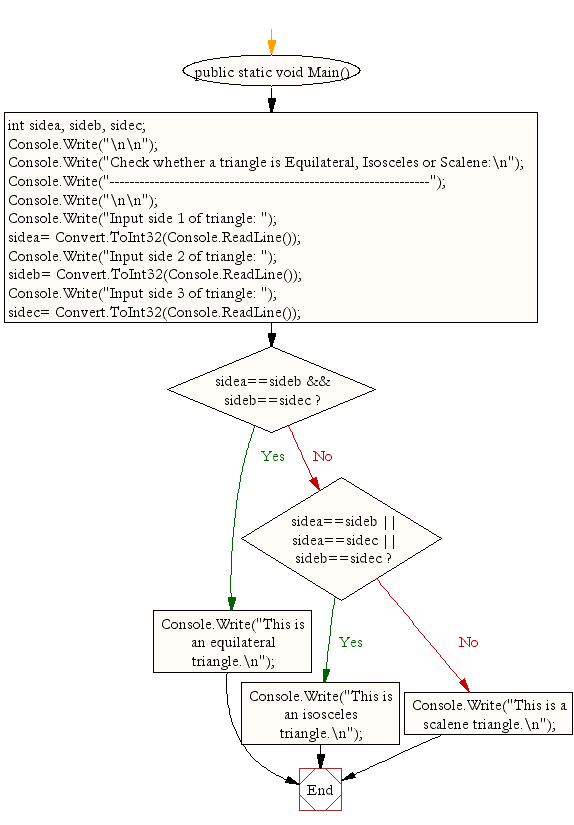
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to read temperature in centigrade and display suitable message according to temperature.
Next: Write a C# Sharp program to check whether a triangle can be formed by the given value for the angles.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework