C# Sharp exercises: Calculate the total, percentage and division to take marks of three subjects
C# Sharp Conditional Statement : Exercise-12 with Solution
Write a C# Sharp program to read roll no, name and marks of three subjects and calculate the total, percentage and division.
Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
public class Exercise12
{
static void Main(string[] args)
{
double rl,phy,che,ca,total;
double per;
string nm,div;
Console.Write("\n\n");
Console.Write("Calculate the total, percentage and division to take marks of three subjects:\n");
Console.Write("-------------------------------------------------------------------------------");
Console.Write("\n\n");
Console.Write("Input the Roll Number of the student :");
rl = Convert.ToInt32(Console.ReadLine());
Console.Write("Input the Name of the Student :");
nm = Console.ReadLine();
Console.Write("Input the marks of Physics : ");
phy= Convert.ToInt32(Console.ReadLine());
Console.Write("Input the marks of Chemistry : ");
che = Convert.ToInt32(Console.ReadLine());
Console.Write("Input the marks of Computer Application : ");
ca = Convert.ToInt32(Console.ReadLine());
total = phy+che+ca;
per = total/3.0;
if (per>=60)
div="First";
else
if (per<60&&per>=48)
div="Second";
else
if (per<48&&per>=36)
div="Pass";
else
div="Fail";
Console.Write("\nRoll No : {0}\nName of Student : {1}\n",rl,nm);
Console.Write("Marks in Physics : {0}\nMarks in Chemistry : {1}\nMarks in Computer Application : {2}\n",phy,che,ca);
Console.Write("Total Marks = {0}\nPercentage = {1}\nDivision = {2}\n",total,per,div);
}
}
Sample Output:
Calculate the total, percentage and division to take marks of three subjects: ------------------------------------------------------------------------------- Input the Roll Number of the student :10 Input the Name of the Student :john smith Input the marks of Physics : 50 Input the marks of Chemistry : 46 Input the marks of Computer Application : 64 Roll No : 10 Name of Student : john smith Marks in Physics : 50 Marks in Chemistry : 46 Marks in Computer Application : 64 Total Marks = 160 Percentage = 53.3333333333333 Division = Second
Pictorial Presentation:
Flowchart:
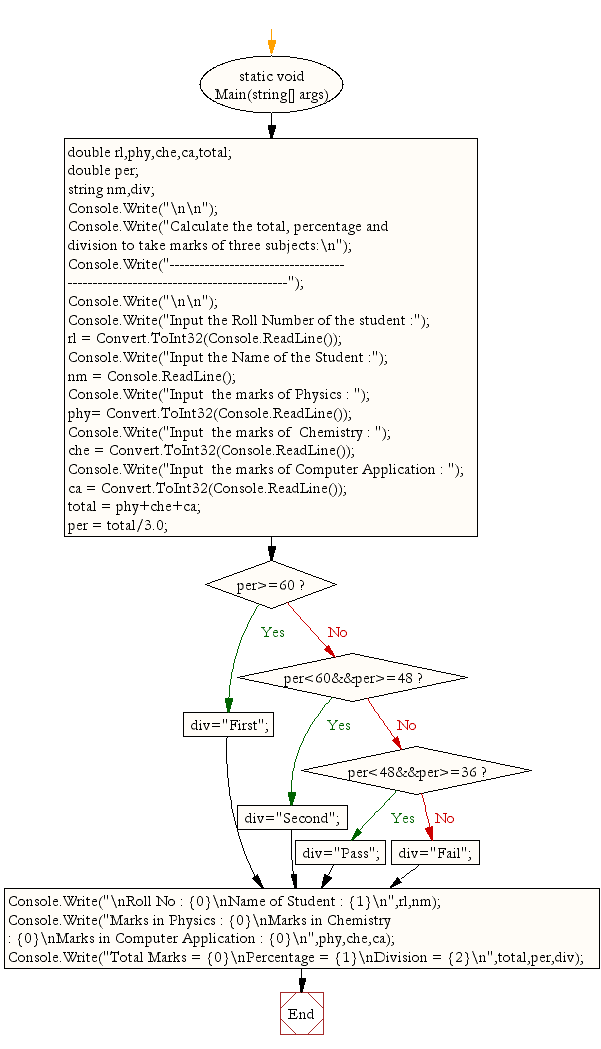
C# Sharp Practice online:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to calculate root of Quadratic Equation.
Next: Write a C# Sharp program to read temperature in centigrade and display suitable message according to temperature.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework