C# Sharp Exercises: Convert all the values of a given array of mixed values to string values
C# Sharp Basic: Exercise-80 with Solution
Write a C# Sharp program to convert all the values of a given array of mixed values to string values.
Sample Solution:
C# Sharp Code:
using System;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
object[] mixedArray = new object[5];
mixedArray[0] = 25;
mixedArray[1] = "Anna";
mixedArray[2] = false;
mixedArray[3] = System.DateTime.Now;
mixedArray[4] = 112.22;
Console.WriteLine("Printing original array elements and their types:");
for (int i = 0; i < mixedArray.Length; i++)
{
Console.WriteLine("Value-> " + mixedArray[i] + " :: Type-> " + mixedArray[i].GetType());
}
string[] new_nums = test(mixedArray);
Console.WriteLine("\nPrinting array elements and their types:");
for (int i = 0; i < new_nums.Length; i++)
{
Console.WriteLine("Value-> "+new_nums[i]+" :: Type-> "+new_nums[i].GetType());
}
}
public static string[] test(object[] nums)
{
return Array.ConvertAll(nums, x => x.ToString());
}
}
}
Sample Output:
Printing original array elements and their types: Value-> 25 :: Type-> System.Int32 Value-> Anna :: Type-> System.String Value-> False :: Type-> System.Boolean Value-> 4/15/2021 10:37:47 AM :: Type-> System.DateTime Value-> 112.22 :: Type-> System.Double Printing array elements and their types: Value-> 25 :: Type-> System.String Value-> Anna :: Type-> System.String Value-> False :: Type-> System.String Value-> 4/15/2021 10:37:47 AM :: Type-> System.String Value-> 112.22 :: Type-> System.String
Flowchart:
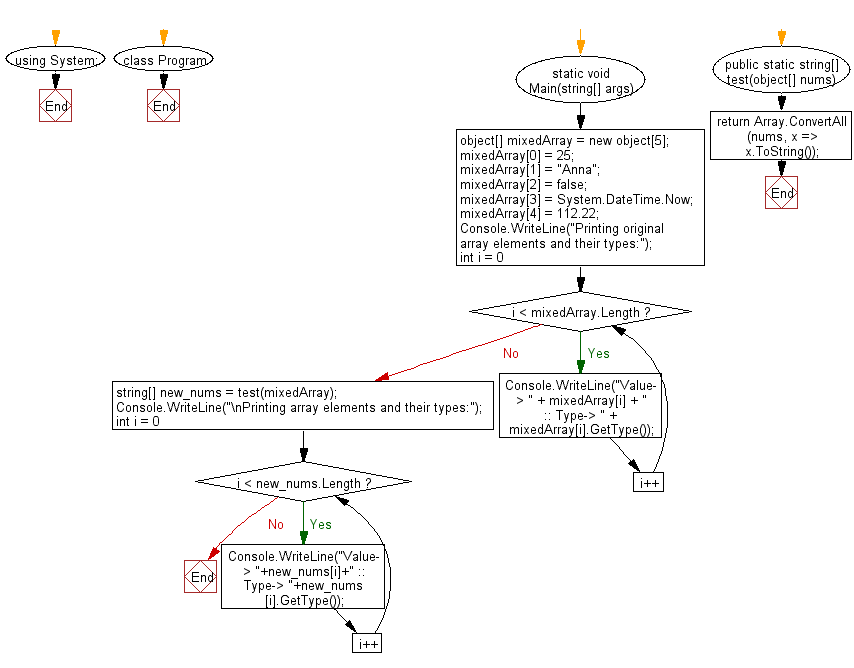
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to convert an integer to string and a string to an integer.
Next: Write a C# Sharp program to swap a two digit given number and check whether the given number is greater than its swap value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework