C# Sharp Exercises: Multiply all of elements of a given array of numbers by the array length
C# Sharp Basic: Exercise-65 with Solution
Write a C# Sharp program to multiply all of elements of a given array of numbers by the array length.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
int[] nums = { 1, 3, 5, 7, 9 };
int[] new_nums = test(nums);
Array.ForEach(new_nums, Console.WriteLine);
}
public static int[] test(int[] nums)
{
var arr_len = nums.Length;
return nums.Select(el => el * arr_len).ToArray();
}
}
}
Sample Output:
5 15 25 35 45
Flowchart:
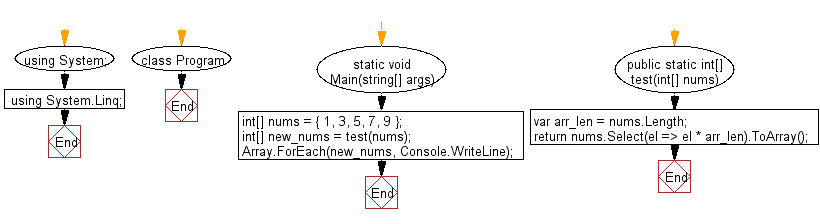
Sample Solution-1:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
int[] nums = { 1, 3, 5, 7, 9 };
int[] new_nums = test(nums);
Array.ForEach(new_nums, Console.WriteLine);
}
public static int[] test(int[] nums)
{
for (int i = 0; i < nums.Length; i++)
{
nums[i] *= nums.Length;
}
return nums;
}
}
}
Sample Output:
5 15 25 35 45
Flowchart:
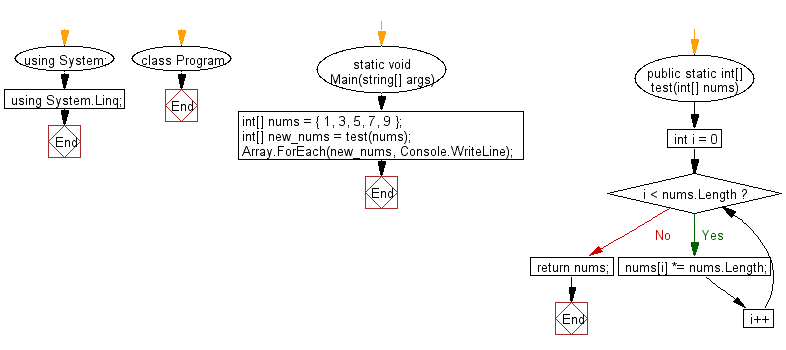
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to get the file name (including extension) from a given path.
Next: Write a C# Sharp program to find the minimum value from two given two numbers, represented as string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework