C# Sharp Exercises: Sort the integers in ascending order without moving the number -5
C# Sharp Basic: Exercise-61 with Solution
Write a C# program to sort the integers in ascending order without moving the number -5.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
using System.Collections;
public class Example
{
public static int[] sort_numbers(int[] arra)
{
int[] num = arra.Where(x => x != -5).OrderBy(x => x).ToArray();
int ctr = 0;
return arra.Select(x => x >= 0 ? num[ctr++] : -5).ToArray();
}
public static void Main()
{
int[] x = sort_numbers(new int[] {-5, 236, 120, 70, -5, -5, 698, 280 });
foreach(var item in x)
{
Console.WriteLine(item.ToString());
}
}
}
Sample Output:
-5 70 120 236 -5 -5 280 698
Flowchart:
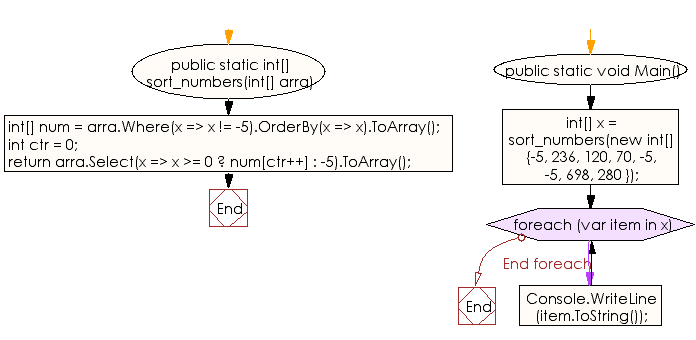
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# program to calculate the sum of all the intgers of a rectangular matrix except those integers which are located below an intger of value 0.
Next: Write a C# program to reverse the strings contained in each pair of matching parentheses in a given string and also remove the parentheses within the given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework