C# Sharp Exercises: Checks how many integers are needed to complete the range
C# Sharp Basic: Exercise-58 with Solution
Write a C# program which will accept a list of integers and checks how many integers are needed to complete the range.
For example [1, 3, 4, 7, 9], between 1-9 -> 2, 5, 6, 8 are not present in the list. So output will be 4.
Sample Solution:
C# Sharp Code:
using System;
public class Example
{
public static int consecutive_array(int[] input_Array)
{
Array.Sort(input_Array);
int ctr = 0;
for(int i = 0; i < input_Array.Length - 1; i++){
ctr += input_Array[i+1] - input_Array[i] - 1;
}
return ctr;
}
public static void Main()
{
Console.WriteLine(consecutive_array(new int[] {1,3, 5,6,9}));
Console.WriteLine(consecutive_array(new int[] {0,10}));
}
}
Sample Output:
4 9
Flowchart:
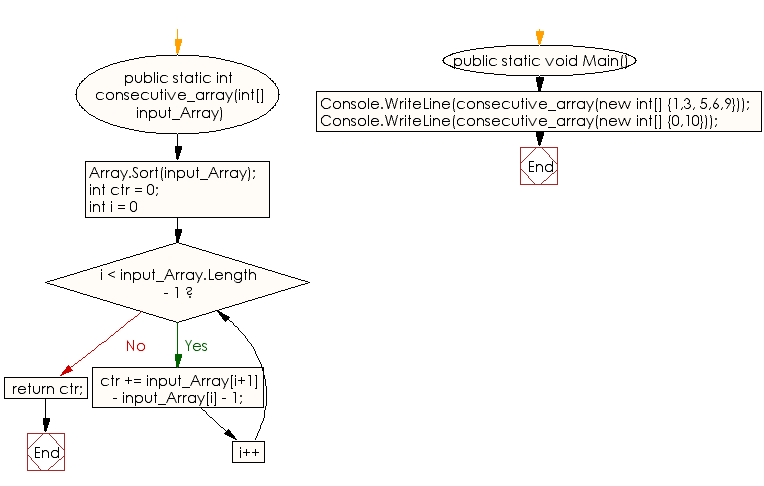
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# program to find the pair of adjacent elements that has the highest product of an given array of integers.
Next: Write a C# program to check whether it is possible to create a strictly increasing sequence from a given sequence of integers as an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework