C# Sharp Exercises: Check two given integers and return true if one is negative and one is positive
C# Sharp Basic: Exercise-18 with Solution
Write a C# program to check two given integers and return true if one is negative and one is positive.
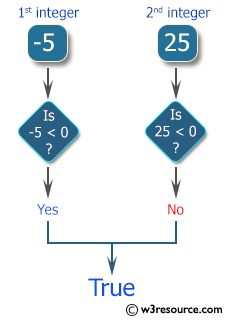
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
public class Exercise18 {
static void Main(string[] args)
{
Console.WriteLine("\nInput first integer:");
int x = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Input second integer:");
int y = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Check if one is negative and one is positive:");
Console.WriteLine((x < 0 && y > 0) || (x > 0 && y < 0));
}
}
Sample Output:
Input first integer: -5 Input second integer: 25 Check if one is negative and one is positive: True
Flowchart:
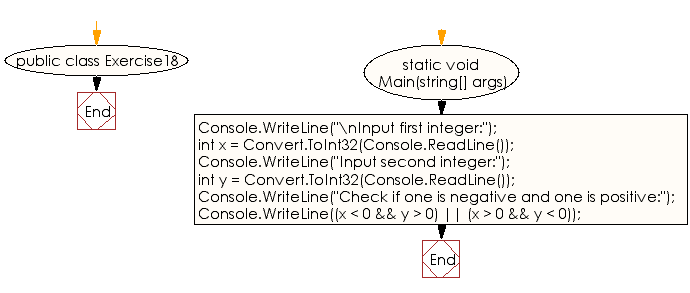
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# program to create a new string from a given string (length 1 or more ) with the first character added at the front and back.
Next: Write a C# program to compute the sum of two given integers, if two values are equal then return the triple of their sum.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework