C# Sharp Basic Algorithm Exercises: Check two given integers and return the value whichever value is nearest to 13 without going over
C# Sharp Basic Algorithm: Exercise-58 with Solution
Write a C# Sharp program to check two given integers and return the value whichever value is nearest to 13 without going over. Return 0 if both numbers go over.
Pictorial Presentation:
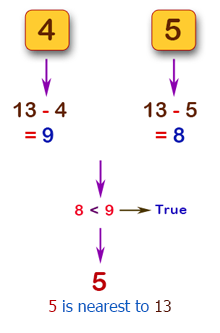

Sample Solution:-
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine(test(4, 5));
Console.WriteLine(test(7, 12));
Console.WriteLine(test(10, 13));
Console.WriteLine(test(17, 33));
Console.ReadLine();
}
public static int test(int x, int y)
{
if (x > 13 && y > 13) return 0;
if (x <= 13 && y > 13) return x;
if (y <= 13 && x > 13) return y;
return x > y ? x : y;
}
}
}
Sample Output:
5 12 13 0
Flowchart:
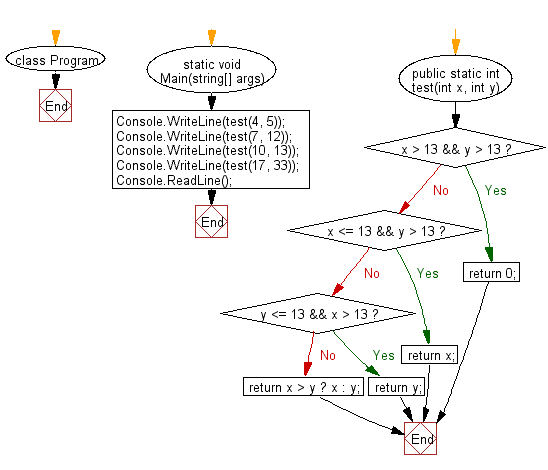
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compute the sum of the three given integers. However, if any of the values is in the range 10..20 inclusive then that value counts as 0, except 13 and 17.
Next: Write a C# Sharp program to check three given integers (small, medium and large) and return true if the difference between small and medium and the difference between medium and large is same.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework