C# Sharp Basic Algorithm Exercises: Create a new list from a given list of integers where each integer multiplied by itself three times
C# Sharp Basic Algorithm: Exercise-142 with Solution
Write a C# Sharp program to create a new list from a given list of integers where each integer multiplied by itself three times.
Pictorial Presentation:
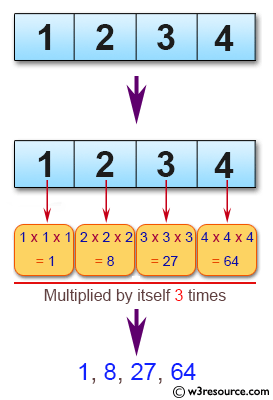
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
List<int> mylist = test(new List<int>(new int[] { 1, 2, 3 , 4 }));
foreach(var i in mylist)
{
Console.Write(i.ToString()+" ");
}
}
public static List<int> test(List<int> nums)
{
IEnumerable<int> squared = nums.Select(x => x * x * x);
return squared.ToList();
}
}
}
Sample Output:
1 8 27 64
Flowchart:
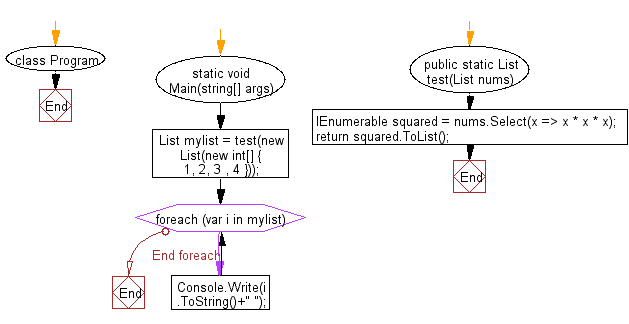
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new list from a given list of integers where each element is multiplied by 3.
Next: Write a C# Sharp program to create a new list from a given list of strings where each element has "#" added at the beginning and end position.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework