C# Sharp Exercises: Find maximum and minimum element in an array
C# Sharp Array: Exercise-9 with Solution
Write a program in C# Sharp to find maximum and minimum element in an array.
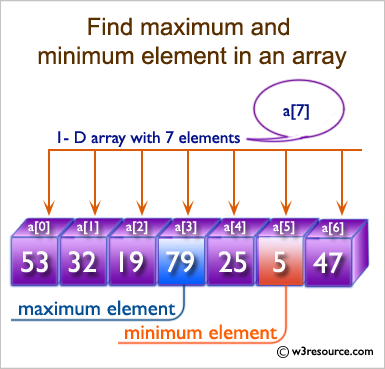
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise9
{
public static void Main()
{
int[] arr1= new int[100];
int i, mx, mn, n;
Console.Write("\n\nFind maximum and minimum element in an array :\n");
Console.Write("--------------------------------------------------\n");
Console.Write("Input the number of elements to be stored in the array :");
n= Convert.ToInt32(Console.ReadLine());
Console.Write("Input {0} elements in the array :\n",n);
for(i=0;i<n;i++)
{
Console.Write("element - {0} : ",i);
arr1[i] = Convert.ToInt32(Console.ReadLine());
}
mx = arr1[0];
mn = arr1[0];
for(i=1; i<n; i++)
{
if(arr1[i]>mx)
{
mx = arr1[i];
}
if(arr1[i]<mn)
{
mn = arr1[i];
}
}
Console.Write("Maximum element is : {0}\n", mx);
Console.Write("Minimum element is : {0}\n\n", mn);
}
}
Sample Output:
Find maximum and minimum element in an array : -------------------------------------------------- Input the number of elements to be stored in the array :2 Input 2 elements in the array : element - 0 : 20 element - 1 : 25 Maximum element is : 25 Minimum element is : 20
Flowchart:

C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to count the frequency of each element of an array.
Next: Write a program in C# Sharp to separate odd and even integers in separate arrays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework