C# Sharp Exercises: Print all unique elements of an array
C# Sharp Array: Exercise-6 with Solution
Write a program in C# Sharp to print all unique elements in an array.
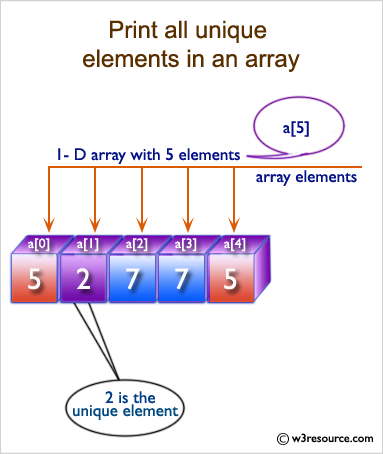
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise6
{
public static void Main()
{
int n,ctr=0;
int[] arr1 = new int[100];
int i, j, k;
Console.Write("\n\nPrint all unique elements of an array:\n");
Console.Write("------------------------------------------\n");
Console.Write("Input the number of elements to be stored in the array :");
n = Convert.ToInt32(Console.ReadLine());
Console.Write("Input {0} elements in the array :\n",n);
for(i=0;i<n;i++)
{
Console.Write("element - {0} : ",i);
arr1[i] = Convert.ToInt32(Console.ReadLine());
}
/*Checking duplicate elements in the array */
Console.Write("\nThe unique elements found in the array are : \n");
for(i=0; i<n; i++)
{
ctr=0;
/*Check duplicate bifore the current position and
increase counter by 1 if found.*/
for(j=0; j<i-1; j++)
{
/*Increment the counter when the seaarch value is duplicate.*/
if(arr1[i]==arr1[j])
{
ctr++;
}
}
/*Check duplicate after the current position and
increase counter by 1 if found.*/
for(k=i+1; k<n; k++)
{
/*Increment the counter when the seaarch value is duplicate.*/
if(arr1[i]==arr1[k])
{
ctr++;
}
/* Duplicate numbers next to each other*/
if(arr1[i]==arr1[i+1])
{
i++;
}
}
/*Print the value of the current position of the array as unique value
when counter remain contains its initial value.*/
if(ctr==0)
{
Console.Write("{0} ",arr1[i]);
}
}
Console.Write("\n\n");
}
}
Sample Output:
Print all unique elements of an array: ------------------------------------------ Input the number of elements to be stored in the array :2 Input 2 elements in the array : element - 0 : 2 element - 1 : 4 The unique elements found in the array are : 2 4
Flowchart:
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to count a total number of duplicate elements in an array.
Next: Write a program in C# Sharp to merge two arrays of same size sorted in ascending order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework