C# Sharp Exercises: Remove all duplicate elements from a given array
C# Sharp Array: Exercise-33 with Solution
Write a C# Sharp program to remove all duplicate elements from a given array and returns a new array.
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
object[] mixedArray = new object[8];
mixedArray[0] = 25;
mixedArray[1] = "Anna";
mixedArray[2] = false;
mixedArray[3] = 25;
mixedArray[4] = System.DateTime.Now;
mixedArray[5] = 112.22;
mixedArray[6] = "Anna";
mixedArray[7] = false;
Console.WriteLine("Original array elements:");
for (int i = 0; i < mixedArray.Length; i++)
{
Console.WriteLine(mixedArray[i]);
}
object[] result = test(mixedArray);
Console.WriteLine("\nAfter removing duplicate elements from the said array:");
for (int i = 0; i < result.Length; i++)
{
Console.WriteLine(result[i]);
}
}
public static object[] test(object[] mixedArray)
{
return mixedArray.Distinct().ToArray();
}
}
}
Sample Output:
Original array elements: 25 Anna False 25 4/15/2021 12:10:51 PM 112.22 Anna False After removing duplicate elements from the said array: 25 Anna False 4/15/2021 12:10:51 PM 112.22
Flowchart:
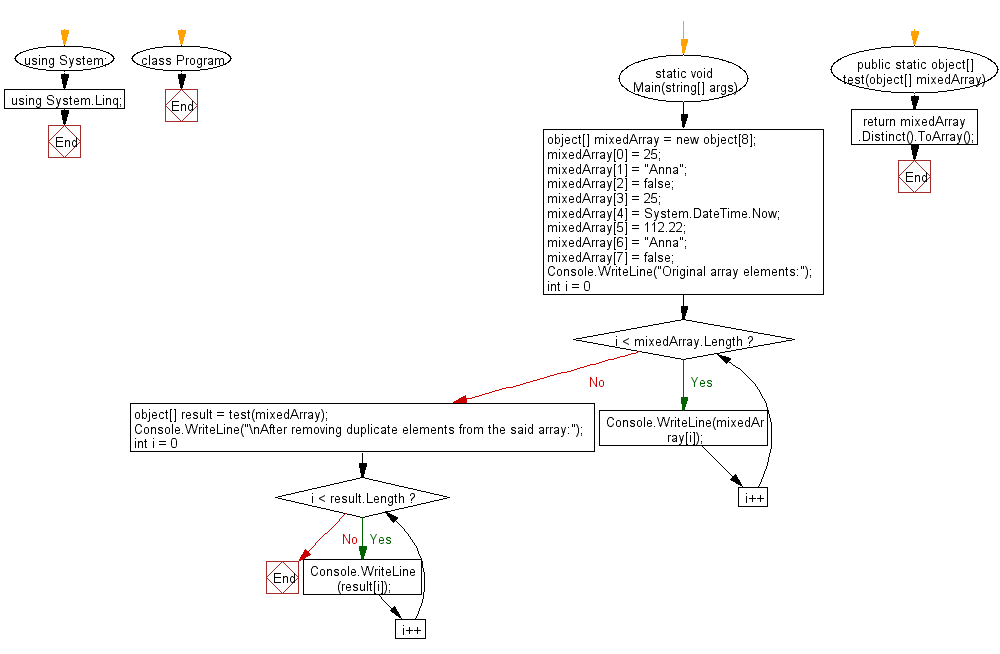
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to get only the odd values from a given array of integers.
Next: Write a C# Sharp program to find the missing number in a given array of numbers between 10 and 20.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework