C# Sharp Exercises: Read n number of values in an array and display it in reverse order
C# Sharp Array: Exercise-2 with Solution
Write a program in C# Sharp to read n number of values in an array and display it in reverse order.
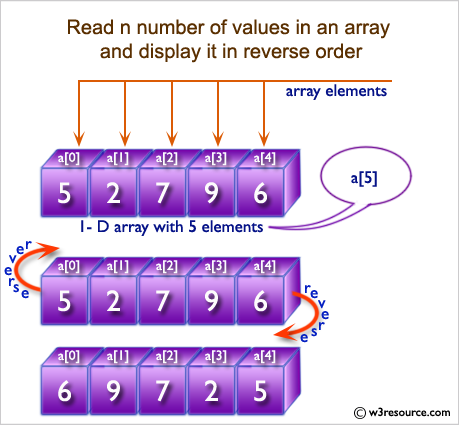
Sample Solution:-
C# Sharp Code:
using System;
public class Exercise2
{
public static void Main()
{
int i,n;
int[] a= new int[100];
Console.Write("\n\nRead n number of values in an array and display it in reverse order:\n");
Console.Write("------------------------------------------------------------------------\n");
Console.Write("Input the number of elements to store in the array :");
n = Convert.ToInt32(Console.ReadLine());
Console.Write("Input {0} number of elements in the array :\n",n);
for(i=0;i<n;i++)
{
Console.Write("element - {0} : ",i);
a[i] = Convert.ToInt32(Console.ReadLine());
}
Console.Write("\nThe values store into the array are : \n");
for(i=0;i<n;i++)
{
Console.Write("{0} ",a[i]);
}
Console.Write("\n\nThe values store into the array in reverse are :\n");
for(i=n-1;i>=0;i--)
{
Console.Write("{0} ",a[i]);
}
Console.Write("\n\n");
}
}
Sample Output:
Read n number of values in an array and display it in reverse order: ------------------------------------------------------------------------ Input the number of elements to store in the array :3 Input 3 number of elements in the array : element - 0 : 1 element - 1 : 2 element - 2 : 3 The values store into the array are : 1 2 3 The values store into the array in reverse are : 3 2 1
Flowchart:
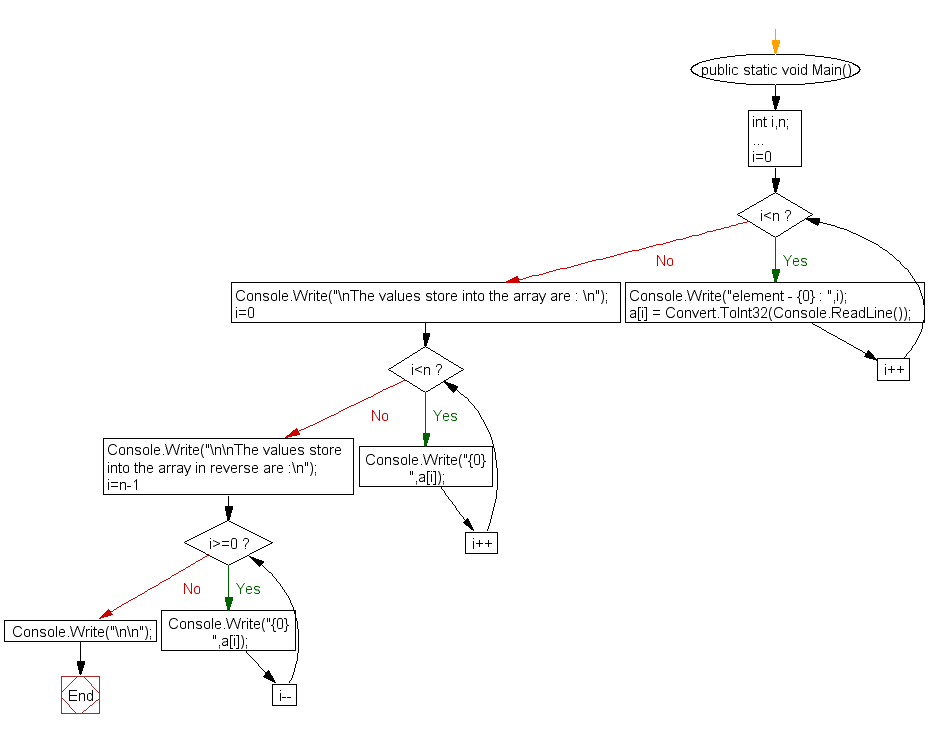
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to store elements in an array and print it.
Next: Write a program in C# Sharp to find the sum of all elements of array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework