C++ Exercises: Sort characters in a string
C++ String: Exercise-5 with Solution
Write a C++ program to sort characters (numbers and punctuation symbols are not included) in a string.
Pictorial Presentation:
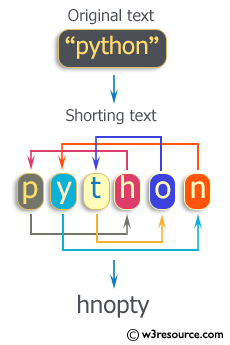
Sample Solution:
C++ Code :
#include <iostream>
#include <string>
using namespace std;
string sort_characters(string text) {
bool flag;
char ch;
do
{
flag = false;
for (int x = 0; x < text.length() - 1; x++)
{
if (text[x] > text[x + 1])
{
ch = text[x];
text[x] = text[x + 1];
text[x + 1] = ch;
flag = true;
}
}
} while (flag);
// Remove spaces
string str;
for (int y = 0; y < text.length(); y++)
{
if (text[y] != ' ')
{
str.push_back(text[y]);
}
}
return str;
}
int main() {
cout << "Original text: python \nSorted text: ";
cout << sort_characters("python") << endl;
cout << "\nOriginal text: AaBb \nSorted text: ";
cout << sort_characters("AaBb") << endl;
cout << "\nOriginal text: the best way we learn anything is by practice and exercise questions \nSorted text: ";
cout << sort_characters("the best way we learn anything is by practice and exercise questions") << endl;
return 0;
}
Sample Output:
Original text: python Sorted text: hnopty Original text: AaBb Sorted text: ABab
Flowchart:

C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find the largest word in a given string.
Next: Write a C++ program to check whether the characters e and g are separated by exactly 2 places anywhere in a given string at least once.
What is the difficulty level of this exercise?