C++ Exercises: Check if a given string is a Palindrome or not
C++ String: Exercise-10 with Solution
Write a C++ program to check if a given string is a Palindrome or not.
A palindrome is a word, number, phrase, or other sequence of characters which reads the same backward as forward, such as madam, racecar.
Pictorial Presentation:
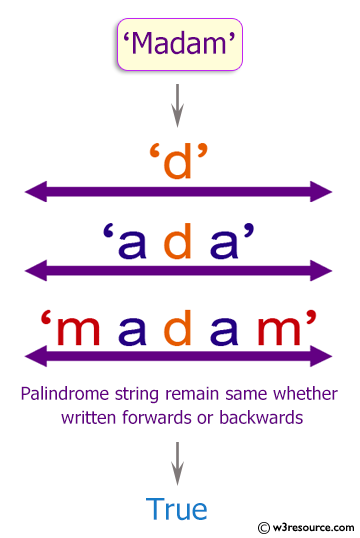
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
string test_Palindrome(string text) {
string str1, str2;
int str_len = int(text.size());
for (int i = 0; i < str_len; i++){
if((text[i] >= 'a' && text[i] <= 'z' ) || (text[i] >= 'A' && text[i] <= 'Z' ))
str1+=tolower(text[i]);
if((text[str_len-1-i] >= 'a' && text[str_len-1-i] <= 'z' ) || (text[str_len-1-i] >= 'A' && text[str_len-1-i] <= 'Z' ))
str2+=tolower(text[str_len-1-i]);
}
if (str1 == str2)
return "True";
return "False";
}
int main() {
cout << "Is madam a Palindrome? " << test_Palindrome("madam");
cout << "\nIs racecar a Palindrome? " << test_Palindrome("racecar");
cout << "\nIs abc a Palindrome? " << test_Palindrome("abc");
return 0;
}
Sample Output:
Is madam a Palindrome? True Is racecar a Palindrome? True Is abc a Palindrome? False
Flowchart:
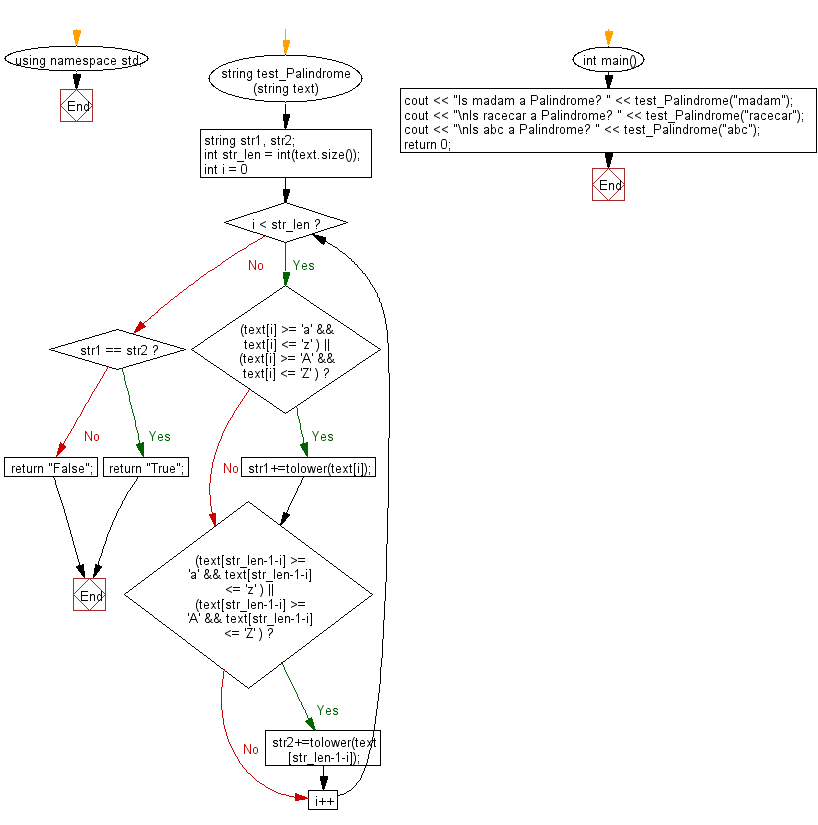
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check whether two characters present equally in a given string.
Next: Write a C++ program to find a word in a given string which has the highest number of repeated letters.
What is the difficulty level of this exercise?