C++ Exercises: Check two numbers are Amicable numbers or not
C++ Numbers: Exercise-28 with Solution
Write a program in C++ to check two numbers are Amicable numbers or not.
Sample Solution:
C++ Code :
#include <bits/stdc++.h>
using namespace std;
int ProDivSum(int n)
{
int sum = 1;
for (int i=2; i<=sqrt(n); i++)
{
if (n%i == 0)
{
sum += i;
if (n/i != i)
sum += n/i;
}
}
return sum;
}
bool chkAmicable(int a,int b)
{
return(ProDivSum(a) == b && ProDivSum(b) == a);
}
int main()
{
int n, i, j, ctr,nm1,nm2;
cout << "\n\n Check whether two numbers are Amicable pairs or not: \n";
cout << "\n Sample: (220, 284), (1184, 1210), (2620, 2924).. \n";
cout << " --------------------------------------------------------\n";
cout<<" Input the 1st number : ";
cin>>nm1;
cout<<" Input the 2nd number : ";
cin>>nm2;
if( chkAmicable(nm1,nm2))
cout << " The given numbers are an Amicable pair."<<endl;
else
cout << " The given numbers are not an Amicable pair."<<endl;
return 0;
}
Sample Output:
Check whether two numbers are Amicable pairs or not: Sample: (220, 284), (1184, 1210), (2620, 2924).. -------------------------------------------------------- Input the 1st number : 220 Input the 2nd number : 284 The given numbers are an Amicable pair.
Flowchart:
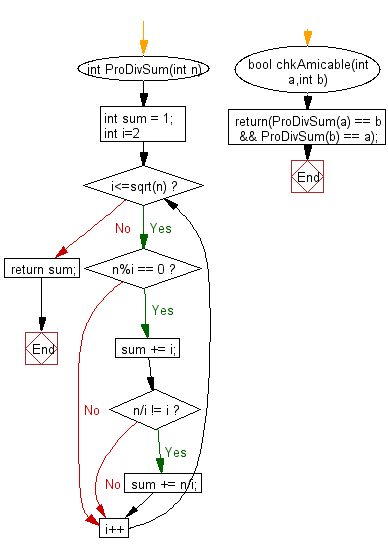
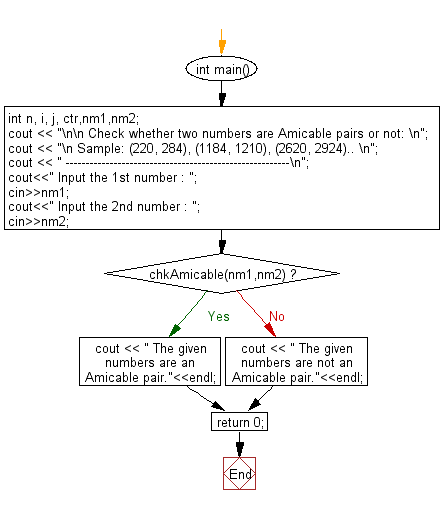
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find Duck Numbers between 1 to 500.
Next: Write a program in C++ to count the Amicable pairs in an array.
What is the difficulty level of this exercise?