C++ Exercises: Find Disarium numbers between 1 to 1000
C++ Numbers: Exercise-19 with Solution
Write a program in C++ to find Disarium numbers between 1 to 1000.
Pictorial Presentation:
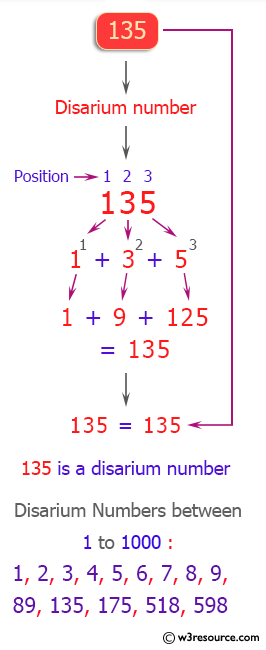
Sample Solution:
C++ Code :
#include<bits/stdc++.h>
using namespace std;
int DigiCount(int n)
{
int ctr_digi = 0;
int tmpx = n;
while (tmpx)
{
tmpx = tmpx/10;
ctr_digi++;
}
return ctr_digi;
}
bool chkDisarum(int n)
{
int ctr_digi = DigiCount(n);
int s = 0;
int x = n;
int pr;
while (x)
{
pr = x % 10;
s = s + pow(pr, ctr_digi--);
x = x/10;
}
return (s == n);
}
int main()
{
int i;
cout << "\n\n Find Disarium Numbers between 1 to 1000: \n";
cout << " ---------------------------------------------\n";
cout << " The Disarium numbers are: "<<endl;
for(i=1;i<=1000;i++)
{
if( chkDisarum(i))
cout <<i<<" ";
}
cout <<endl;
}
Sample Output:
Find Disarium Numbers between 1 to 1000: --------------------------------------------- The Disarium numbers are: 1 2 3 4 5 6 7 8 9 89 135 175 518 598
Flowchart:
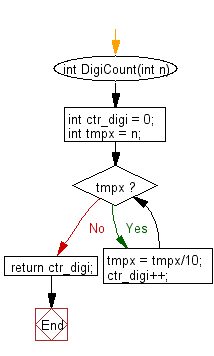
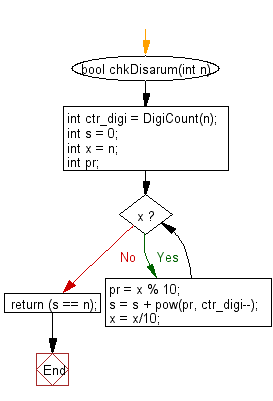
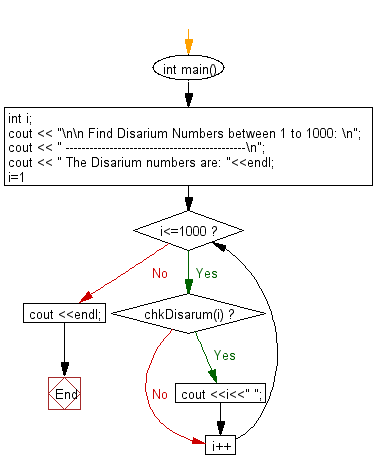
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to check whether a number is Disarium or not.
Next: Write a program in C++ to check if a number is Harshad Number or not.
What is the difficulty level of this exercise?