C++ Exercises: Calculate x raised to the power n
C++ Math: Exercise-5 with Solution
Write a C++ program to calculate x raised to the power n (xn).
Sample Input: x = 7.0
n = 2
Sample Output: 49
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
double powxn(double x, int n) {
if (n < 0){
x = 1 / x;
n = -n;
}
double result = 1;
for (auto i = 0; i < n; ++i)
{
result = result * x;
}
return result;
}
int main(void)
{
double x = 7.0;
int n = 2;
cout << "\n" << x << "^" << n << " = " << powxn(x, n) << endl;
x = 3;
n = 9;
cout << "\n" << x << "^" << n << " = " << powxn(x, n) << endl;
x = 6.2;
n = 3;
cout << "\n" << x << "^" << n << " = " << powxn(x, n) << endl;
return 0;
}
Sample Output:
7^2 = 49 3^9 = 19683 6.2^3 = 238.328
Flowchart:
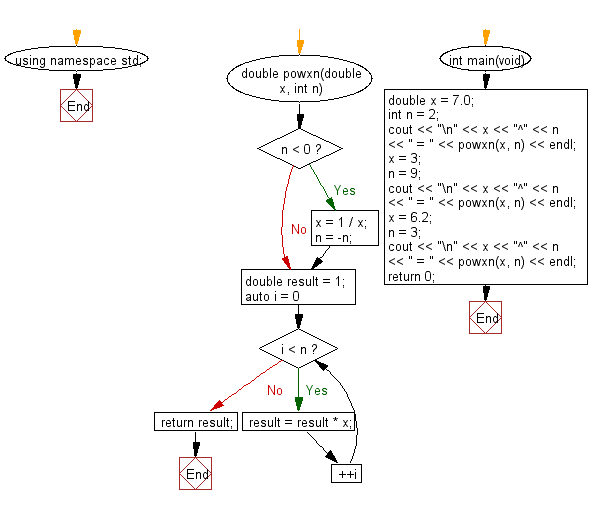
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to divide two integers (dividend and divisor) without using multiplication, division and mod operator.
Next: Write a C++ program to get the fraction part from two given integers representing the numerator and denominator in string format.
What is the difficulty level of this exercise?