C++ Exercises: Count all the numbers with unique digits within a given range
C++ Math: Exercise-29 with Solution
Write a C++ program to count all the numbers with unique digits within a given range 0 ≤ y < 10n where y represent the unique digits numbers and take n as a input from the user.
Sample Input: n = 1
Number of unique digits: 10
Sample Input: n = 2
Number of unique digits: 91
Sample Solution:
C++ Code :
#include <iostream>
#include <cmath>
using namespace std;
int count_Unique_Digits_numbers(int n) {
if (n == 0) {
return 1;
}
int ctr = 10;
for (int k = 2, fk = 9; k <= n; ++k) {
fk *= 10 - (k - 1);
ctr += fk;
}
return ctr;
}
int main()
{
int n = 1;
cout << "\nn = " << n << ", Number of unique digits: " << count_Unique_Digits_numbers(n) << endl;
n = 2;
cout << "\nn = " << n << ", Number of unique digits: " << count_Unique_Digits_numbers(n) << endl;
return 0;
}
Sample Output:
n = 1, Number of unique digits: 10 n = 2, Number of unique digits: 91
Flowchart:
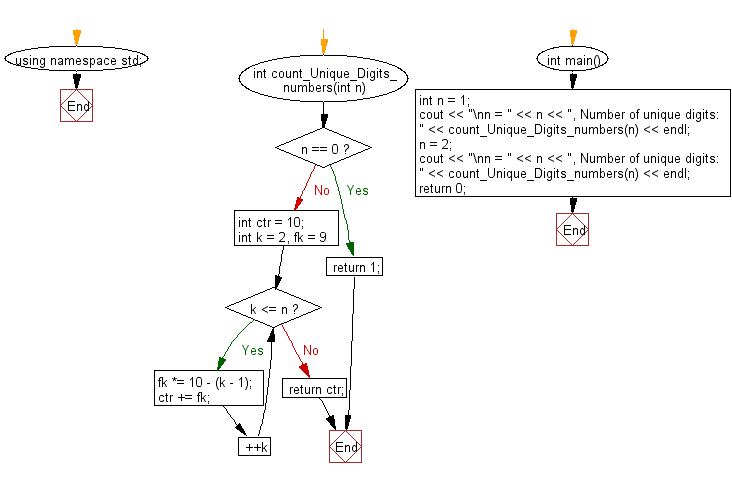
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to break a given integer in at least two parts (positive integers) to maximize the product of those integers.
Next: Write a C++ program to check whether a given positive integer is a perfect square or not.
What is the difficulty level of this exercise?