C++ Exercises: Find the missing number in a given array of integers taken from the sequence 0, 1, 2, 3, ...,n
C++ Math: Exercise-26 with Solution
Write a C++ program to find the missing number in a given array of integers taken from the sequence 0, 1, 2, 3, ...,n.
Sample Input: arr[10] = {10, 9, 4, 6, 3, 2, 5, 7, 1, 0 }
Sample Output: Missing number in the said array:
8
Sample Input: arr1[4] = {0, 3, 4, 2}
Sample Output: Missing number in the said array:
1
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
int missingNumber(int nums[], int arr_size) {
if (NULL == nums || arr_size == 0) {
return 0;
}
int result = arr_size;
for (int i = 0; i < arr_size; i++) {
result ^= i;
result ^= nums[i];
}
return result;
}
int main() {
int arr[10] = {10, 9, 4, 6, 3, 2, 5, 7, 1, 0 };
int arr_size = sizeof(arr) / sizeof(arr[0]);
cout << "Original array\n";
for (int i = arr_size - 1; i >= 0; i--)
cout << arr[i] << " ";
cout << "\nMissing number in the said array: ";
cout << "\n" << missingNumber(arr, arr_size);
int arr1[4] = {0, 3, 4, 2};
arr_size = sizeof(arr1) / sizeof(arr1[0]);
cout << "\n\nOriginal array\n";
for (int i = arr_size - 1; i >= 0; i--)
cout << arr1[i] << " ";
cout << "\nMissing number in the said array: ";
cout << "\n" << missingNumber(arr1, arr_size);
return 0;
}
Sample Output:
Original array 0 1 7 5 2 3 6 4 9 10 Missing number in the said array: 8 Original array 2 4 3 0 Missing number in the said array: 1
Flowchart:
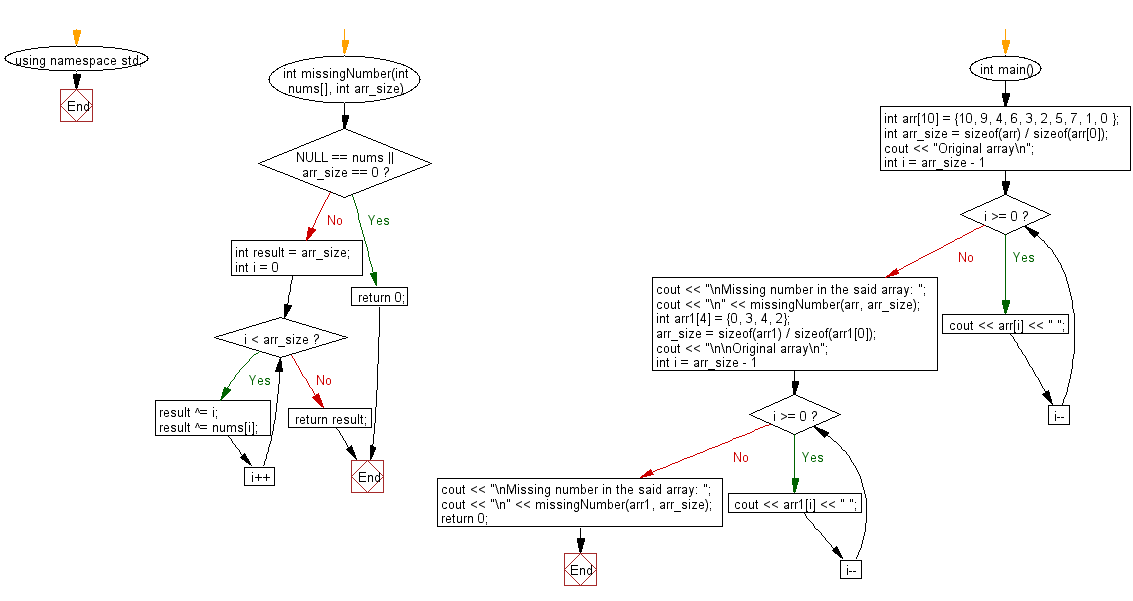
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to count the total number of digit 1 pressent in all positive numbers less than or equal to a given integer.
Next: Write a C++ program to find the number of perfect square (e.g. 1, 4, 9, 16, ...) numbers which represent a sum of a given number.
What is the difficulty level of this exercise?